Please purchase the course to watch this video.
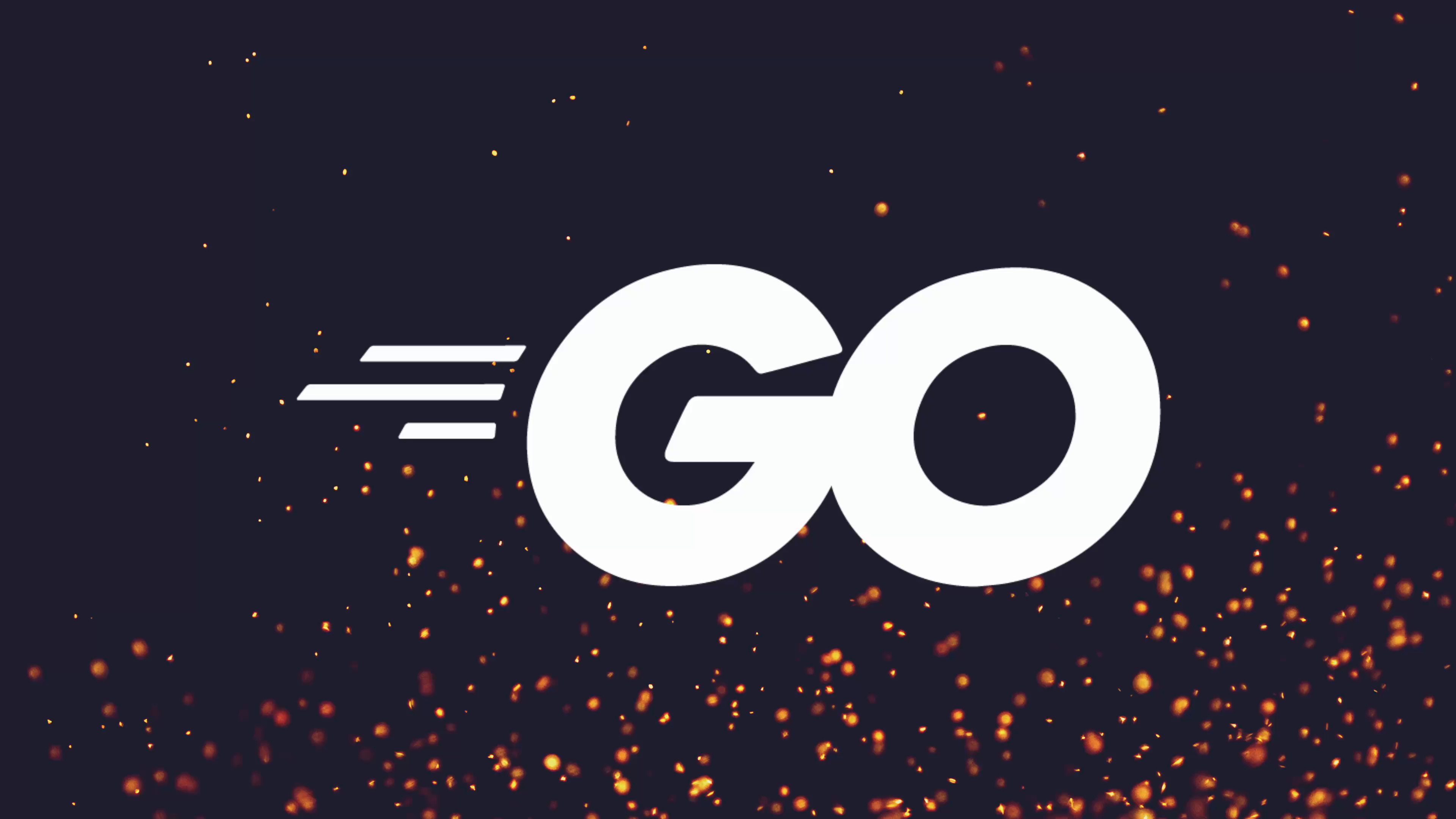
Full Course
Full Course
Managing file operations in programming is crucial for ensuring that applications run smoothly without hitting resource limits. When handling multiple files, it's essential to close them properly after use, a practice known as "always be closing" (A.B.C). The Go programming language provides an effective solution for this using the defer
keyword, which ensures that resources are released even if a function exits prematurely. By deferring the closure of files, developers can prevent issues related to exceeding the maximum number of open files, thus avoiding runtime errors and maintaining cleaner code. This lesson highlights the importance of proper file management, illustrates the utility of the defer
statement in Go, and discusses best practices for error handling when closing files, ultimately leading to more robust and efficient applications.
Now that our application is reading from multiple files, we actually have another issue that we need to resolve. To show what this issue is, let me open up another terminal window and run the sh
command to load the born shell. Then if I go ahead and use the following ulimit
command, similar to what we saw before, however, this time specifying it to the number six, this command will only allow me to open six files at a single time.
Now, if I go ahead and run our counter CLI application, passing in three different files, when I go to run this code, you can see it works for our first file of errors.txt
, however, both the file foo.txt
and words.txt
produce an error. If we take a look at the error, you can see it's telling us that we have too many open files. You may be confused at why opening three different files fails when we've set the limit to six. However, it's worthwhile considering that opening the actual binary itself takes one file, and if you'll remember, each process also has three file streams added to it, standard in, standard out, and standard error. In total, that means we have one file left over, which we can use for opening up the errors.txt
, however, afterwards, we're unable to open either the foo.txt
or words.txt
because we have too many open files.
However, this is actually a bug in our code, as we're not opening each file in parallel, instead opening them one by one. This is because we're not actually closing these files once we're finished reading from them, and haven't been ever since we moved away from the os.ReadFile
function. When it comes to Go and other programming languages, there's a simple golden rule you should always remember. A.B.C. Which stands for always be closing. Specifically, closing open files whenever you're finished with them. By not doing so, it can not only cause your application to stop working once too many files have been opened, but can also impact other processes as well.
Now, to be fair, back when our application was only reading from a single file, whilst it is still bad to not close this file once we're done, it wasn't too much of an issue. This is because any files that have been opened by a process are automatically closed by the operating system when that process exits. However, now that our code is able to open multiple files, we're more likely to run into issues, such as the one we saw before. Additionally, as I mentioned before, it's generally a bad practice to leave files open longer than you need them.
So, in this lesson, we're going to go about rectifying our open file problem, whilst also learning about the defer
keyword. To begin, let's head back on over to our main.go
file, and scroll down to our CountWordsInFile
function. Here is where we're actually opening our file using the Open
function of the os
package. Therefore, this is where we're going to want to close it, specifically after line 51, which is once we're done reading the file.
In order to do so, we can shuffle this code around. First, capturing the count of words in a variable named count
, before replacing our return value to instead return this count
, as well as the nil
error. Then we can go ahead and close the file just underneath this CountWords
function. Whilst this works, it makes the CountWordsInFile
function a little less clear than it was before. Not only this, but if we also happen to have another function on the following line, which also returned an error, then we would also need to make sure that we happen to be closing this file here as well.
Whilst we're not likely going to run into this issue, there is always the potential that we could. And remembering to call the file.Close
method at every early return is something that's likely going to lead to bugs. Fortunately, Go provides a way to make sure that we don't forget to do this, using the defer
keyword. This keyword can be used with any function when it comes to Go, causing that function to be called whenever the parent function returns.
To show the defer
keyword in action, let's go ahead and use it with the following fmt.Println
function, printing out the words, "I am deferred" to the console. Then let's go ahead and add in the following print line statement, letting us know that the file is closed. Now, if I go ahead and open up another terminal window, and use the go run
command, passing in the words.txt
file, you can see here the order of operations is a little different than what you'd expect. The first line that's printed out is the code on line 57, where we're letting the user know that the file is closed. Then afterwards, the print line statement on line 51 happens next, which is the "I am deferred" print line statement.
This is because, as I mentioned, the defer
statement happens after the return value. So if we could write this out in a linear way, the print line statement is actually happening here. Of course, when it comes to programming languages, you can't actually run code after the return statement. However, Go makes this possible by using the defer
keyword. The reason this is so powerful is because if we happen to accidentally return early here, before we actually call the file.Close
function, you can see that the deferred statement is still being called.
This means instead of using the file.Close
manually, we can instead use it on line 51, which guarantees that the file.close
method will be called, even if there's other returns later on. When it comes to Go, using the defer
keyword is the idiomatic approach for calling any functions that need to occur, whether or not the function was successful. This is because not only will they be run after the return statement, but they're also run in the event of a panic.
For example, if I go ahead and replace the file.Close
with an anonymous function, putting in a print line saying "closing file", followed by actually closing it, then if we go ahead and add a panic letting us know that something went wrong. If we go ahead and call this code using the go run
command as follows, you can see we still close the file despite the panic. This is going to be useful later on when we look at adding in concurrency through the use of Go routines, as a panic inside of a Go routine doesn't actually cause the application to crash, only that individual thread.
Therefore, for guaranteeing that anything should happen at the end of the file, it's definitely recommended to use the defer
keyword. Before we move on, one thing you may have noticed is that the close method of the file actually returns an error. If you cast your memory back to earlier on in this course, I mentioned that we should never ever ignore errors. However, in this case, I think it's worth breaking this rule. The reason as to why is due to the fact that we don't actually have any form of recourse when it comes to this error.
For example, if something does go wrong, what's the point of letting the user know? The action of counting the number of words still was correct, and there's no real action that they can take. As I mentioned, the file will be closed by the operating system when the process exits. If we consider the other form of error handling that we have, which is letting the operating system know that something went wrong using a non-zero error code, there's no reason to do this either, as again, the operation to count the number of words was successful, and we're going to be printing out the correct response, regardless of whether or not this file is closed.
Therefore, for this specific use case, it's okay to ignore the error, which you can either do as follows when it comes to using an anonymous function, or you can do so by just calling defer
on the actual method itself. However, if you happen to be writing code where it's still advantageous to actually log the error, you can do so as follows, first capturing it, and then handling it inside of the defer
statement as you would do normally. Just keep in mind, however, you can't actually return the error inside of the deferred statement, as this function is called after the actual return value of its parent function.
Therefore, the only real recourse that you have is to log it out using, say, a logger. Let's go ahead and quickly revert this code so that we're deferring the file.Close
method. Then, if I go ahead and build this code, and open up a new terminal window before setting the same ulimit
that I had before, allowing for only six open files. If I go ahead and execute this code as I did before, passing in three files, you can see this time we no longer get the "too many open files" error, as we did before.
With that, our code is now working as expected. Let's go ahead and commit our changes before moving on to the next lesson.