Please purchase the course to watch this video.
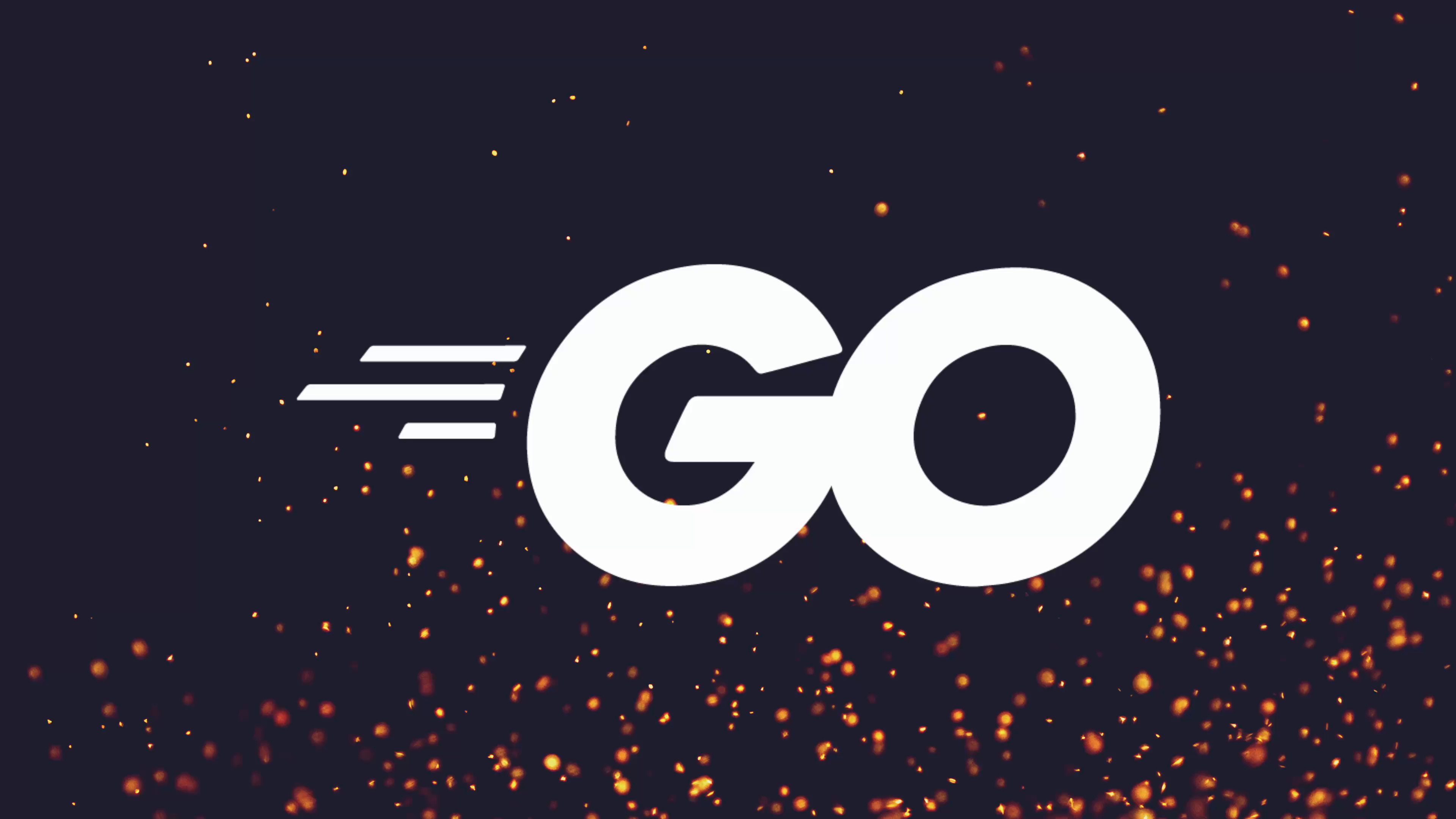
Full Course
End-to-end testing plays a crucial role in ensuring the reliability of file operations in Go applications. This lesson focuses on implementing a test for file behavior, guiding the construction of a function to manage executable commands and handle temporary files effortlessly. Key techniques explored include creating temporary files using the os
package, managing file I/O with proper error handling, and utilizing deferred function calls to maintain correct execution order. Additionally, insights are provided on capturing command outputs and handling errors effectively, allowing developers to streamline their testing process while ensuring that both standard output and error streams behave as expected. These strategies not only simplify testing but also enhance code reliability when dealing with file operations.
No links available for this lesson.
Now that we have an end-to-end test testing standard input, let's go ahead and add in an end-to-end test for testing the file behavior.
Creating the Test Function
We'll create a new test called TestSingleFile
:
func TestSingleFile(t *testing.T) {
We want to reuse the logic that gets the executable path. So let's extract that to a helper function:
func getCommand(args ...string) (*exec.Cmd, error) {
dir, err := os.Getwd()
if err != nil {
return nil, err
}
path := filepath.Join(dir, binName)
return exec.Command(path, args...), nil
}
Back in TestSingleFile
, we can now call:
cmd, err := getCommand()
if err != nil {
t.Fatal("could not get command:", err)
}
Creating a Temporary File
We'll now create a temporary file to pass into our CLI app.
file, err := os.CreateTemp("", "counter-test")
if err != nil {
t.Fatal("couldn't create temp file:", err)
}
defer os.Remove(file.Name()) // Delete file after test
Print the file path to confirm:
t.Logf("Temp file created at: %s", file.Name())
Now we write content to it:
_, err = file.WriteString("foo bar baz\nbaz bar foo\none, two, three")
if err != nil {
t.Fatal("could not write to temp file:", err)
}
if err := file.Close(); err != nil {
t.Fatal("could not close file:", err)
}
Pass the File to the Command
We now pass the filename as an argument:
cmd, err = getCommand(file.Name())
if err != nil {
t.Fatal("could not create command:", err)
}
Capture stdout:
var out bytes.Buffer
cmd.Stdout = &out
Run the command:
if err := cmd.Run(); err != nil {
t.Fatal("failed to run command:", err)
}
Comparing Expected Output
We generate expected output using fmt.Sprintf
:
expected := fmt.Sprintf(" 33 9 3\n")
Compare:
if out.String() != expected {
t.Logf("stdout not correct.\nGot: %q\nWant: %q", out.String(), expected)
t.Fail()
}
Test for Non-Existent File
Now let’s test that the CLI fails gracefully for a file that doesn’t exist.
func TestNoExist(t *testing.T) {
cmd, err := getCommand("no-exist.txt")
if err != nil {
t.Fatal("could not get command:", err)
}
var stderr, stdout bytes.Buffer
cmd.Stderr = &stderr
cmd.Stdout = &stdout
err = cmd.Run()
if err == nil {
t.Log("command succeeded when it should have failed")
t.Fail()
} else if !strings.Contains(err.Error(), "exit status 1") {
t.Logf("expected error to contain 'exit status 1', got: %v", err)
t.Fail()
}
expectedErr := fmt.Sprintf("counter: open no-exist.txt: no such file or directory\n")
if stderr.String() != expectedErr {
t.Logf("stderr not correct.\nGot: %q\nWant: %q", stderr.String(), expectedErr)
t.Fail()
}
if stdout.String() != "" {
t.Logf("stdout should be empty, got: %q", stdout.String())
t.Fail()
}
}
Summary of What We've Done
We’ve now added three E2E tests:
- ✅
TestStdin
— test reading from standard input - ✅
TestSingleFile
— test reading from a single file - ✅
TestNoExist
— test error on non-existent file
You could simplify these with table-driven tests, but we've kept them verbose to see quirks and behavior up close.
Next up: we'll add tests for handling multiple files.