Please purchase the course to watch this video.
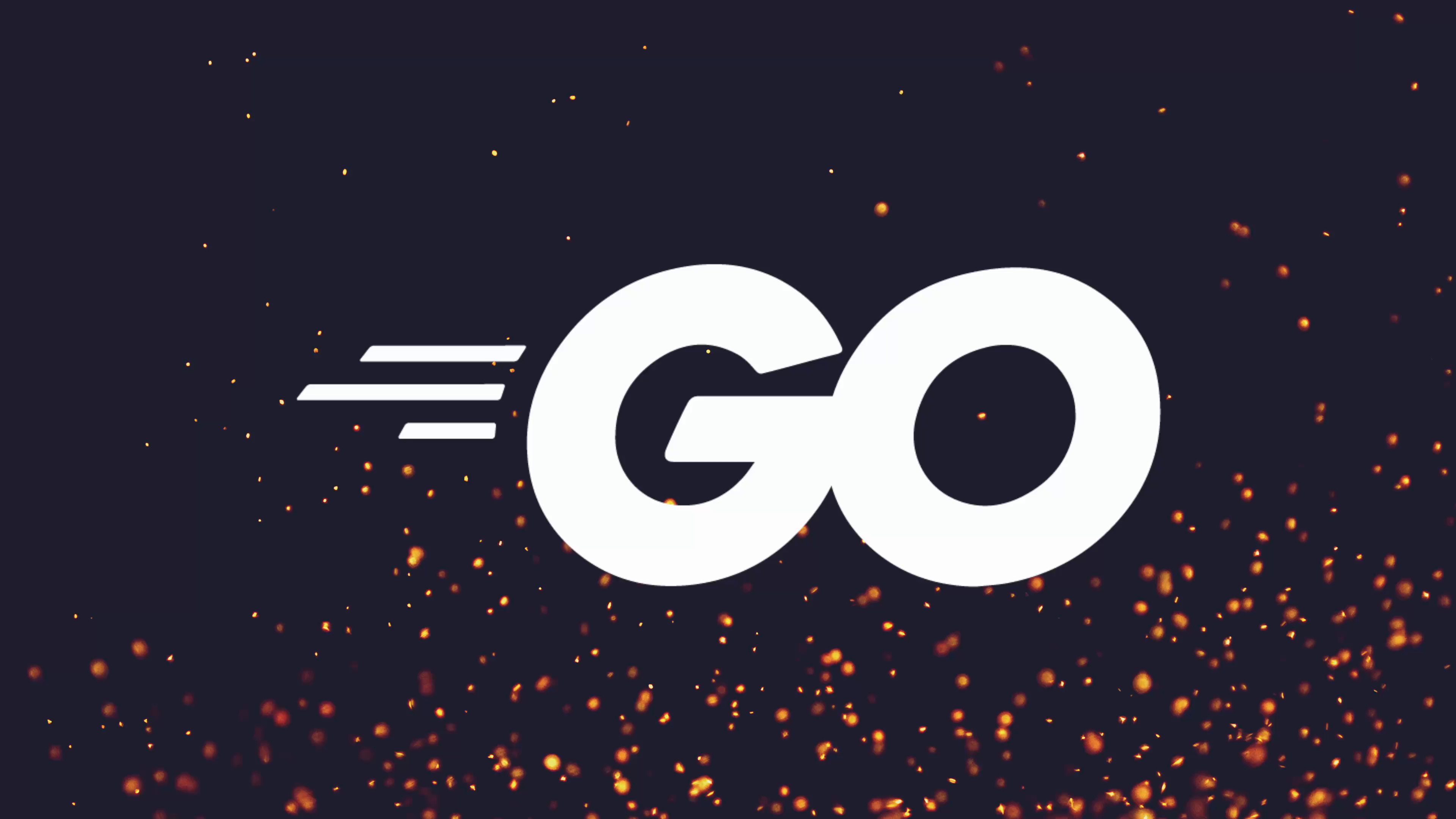
Full Course
Passing input to commands is an essential aspect of command execution in programming, particularly in Go. Two primary methods are used to funnel data into commands: command line arguments and the standard input stream. Command line arguments are passed as unique strings—allowing for effective handling of spaces through automatic escaping—while the standard input stream can be fed data via io.reader
. Utilizing the exec
package's standard in pipe
offers a robust way to stream input into commands, automatically closing the pipe when the command completes. This functionality not only simplifies the process but also integrates seamlessly with various IO
constructs, enabling complex input-output operations such as redirecting inputs to both a command and the standard output simultaneously—enhancing the versatility and power of command execution in applications.
No links available for this lesson.
Now that we know how to capture output from our commands, let's talk about the other side of the equation, passing in input. When it comes to passing in input to commands, you typically have two different approaches. The first is through the use of command line arguments, which we actually saw already when we were using the wc
command, passing in the file we wanted to count, such as words.txt
, which if I go ahead and run, you can see calls the word counter command with the words.txt
argument and produces the correct result.
As we saw in the end-to-end tests, each argument that's passed into our command needs to be its own unique string. This is because the exec
command will do some escaping in the event that a single argument happens to have a space in it. For example, if a filename happens to be mywords.txt
, which can happen but really shouldn't on a Unix system, then the exec.Command
function will automatically escape this space for us.
For example, if I go ahead and change the words.txt
to be my space words.txt
, which is now a file with a space in it, if I go ahead and run the code now, you can see it still manages to print out these words. Therefore, for each individual argument, it's passed in as an individual string, rather than passing in all of the arguments as a single string, in order for the command to be able to escape any spaces that might exist within the actual command itself.
Let's go ahead and revert my words back to words.txt
for the moment. In addition to command line arguments, another form of passing in data is through the use of the standard input stream. We've seen the standard input stream quite a lot throughout this course, so we should have a good understanding of how it works. When it comes to our own applications, the standard input stream is an io
, it is an os.File
, which we can basically read from. When it comes to a command, however, the standard input stream is actually an io.Reader
, which means we can pass in some data via an io.Reader
that will then be read by the command.
We've already seen this behaviour when it came to our end-to-end tests, where we were using the standard input property of a command in order to pass in a strings.Reader
to test the standard input capabilities of our word counter. Or just general counter. To take a look at this in more detail, let's go ahead and use the cat
command. And we'll pass in the standard input stream, and again we'll use a strings.Reader
just because it's easier for us to type for us to type of 123
.
Now if we go ahead and run this code as follows, you can see it prints out 123
. Pretty cool. Because of this, it means we can use standard input with a number of different types, such as an actual os.file
. Let's say we want to go ahead and use the f
, and we'll just ignore the error for the moment.
os.Open("words.txt")
Then we could go ahead and pass this file in as follows. Now if I go ahead and run this code, you can see it prints out the contents of our words.txt
, or perhaps we want to be able to pass in a type to the standard error stream that we can actually write to. For example, such as by creating an io.Pipe()
that we saw before, which if you'll remember, if we go ahead and import it, creates a synchronous in-memory pipe that can be used to connect code expecting an io.Reader
, which our standard input stream is, with code expecting an io.Writer
.
So we could go ahead and create this pipe and pass it in to standard inputs, then we could just go ahead and write whatever we want to the io.Pipe
. For example, we could go ahead and wrap a go
function where we pass in our own standard input stream, although it probably would be simpler to just pass this in directly. But we could do an io.Copy
to the pipe writer, and we'll just do
os.Stdin
in lieu of any other examples. We need to go ahead and actually close the piped writer. Now if we go ahead and run this code, we should be able to do 1, 2, 3, 4, 5
perhaps. And if we Ctrl + D
, it just echoes everything that we wrote to it.
Whilst using io.Pipe()
is one approach to have a piped reader writer so that we can write data to a command's standard input stream, it's not actually the approach I would recommend. This is because the command type of the exec
package has a method called StdinPipe()
, which returns a pipe that will be connected to the command's standard input when the command starts. Not only this, but it also has some additional features such as automatically closing once the command waits for the command to exit, so in general it's slightly more featured than using a typical io.Pipe()
.
Therefore, let's take a quick minute to see how this pipe actually works. To take a look at how this works, rather than using the cat
command, which we saw already, let's instead go ahead and call the wc
command as follows. By default, wc
will accept standard input, which we can pipe in 1, 2, 3
, and if we close using Ctrl + D
, it will produce the output. So let's go ahead and use this as follows.
We can call the exec
command, and then rather than passing in an io.Reader
to the standard input stream, let's instead go ahead and call the StdinPipe()
. In order to get the actual pipe, which we'll call as w
because it's a writer, we'll call it as pipe
, and we'll get the error as well. Then we'll do a simple if
error check, and we'll just return early and then print failed to get pipe
, as follows:
if err != nil {
return fmt.Errorf("failed to get pipe: %w", err)
}
Now we can go ahead and write to this pipe using, say, the Write
function, in which case we can go ahead and pass in a slice of bytes of 1, 2, 3, 4, 5
, and then we can just go ahead and close it using pipe.Close()
. Now if we go ahead and run the code, we can see it works as it did before.
Let's go ahead and pass in a new line as well, I think, just to make this a little easier. There we go, we can see it works as it did before. However, this time we're using an io.Writer
rather than passing in an io.Reader
. This means we can actually do some rather interesting things, especially when it comes to using our existing io
constructs that we saw before.
For example, if we take a look at the MultiWriter
that we saw before, io.MultiWriter
, we can pass in our pipe and maybe even os.Stdout
. Then we could just go ahead and capture this as follows, and we could do a
w.Write
and we'll just write the same thing. Then we'll make sure to close the existing pipe so the application can exit. Now if we run this code, you can see we print to both standard outputs, and we're also printing to the wc
command where we're getting the result back from later on.
As you can see, by coupling the standard input pipe with other io
constructs, it means you can do some very interesting things, and your imagination is really only the limit. For example, let's say we want to pipe our own standard inputs to both the subcommand and also to standard out as well. We could do that by using the TeeReader
that we saw before. So if we go ahead and use r
and we do the io.TeeReader
, in which case we'll read from os.Stdin
, and we can write to our pipe, let's say.
Then we could go ahead and just do a simple io.Copy
to os.Stdout
, and we can copy from the reader. Now if I go ahead and run this code, you'll see that we're waiting for standard input to come in. So hello world
, and you'll see we passed, we already piped it to standard out. And if I go ahead and close this, you can see we're then also passing it in to our wc
command. So you could do things like this line will be printed and then counted once complete. This line will also be. Now if we go ahead and close the stream using Ctrl + D
, you can see we get a running total of all of the words that we entered in.
With that, we've managed to take a look at how we can pass input to our subcommands through the use of the standard input property, as well as through the use of the standard input pipe, which provides us an io.WriteCloser
in order to be able to write data to our subprocess. In the next lesson, we're going to take a look at how we can configure our processes even further, before then moving on to asynchronous processes where we use the other two pipes available to us - the standard output pipe and the standard error pipe.