Please purchase the course to watch this video.
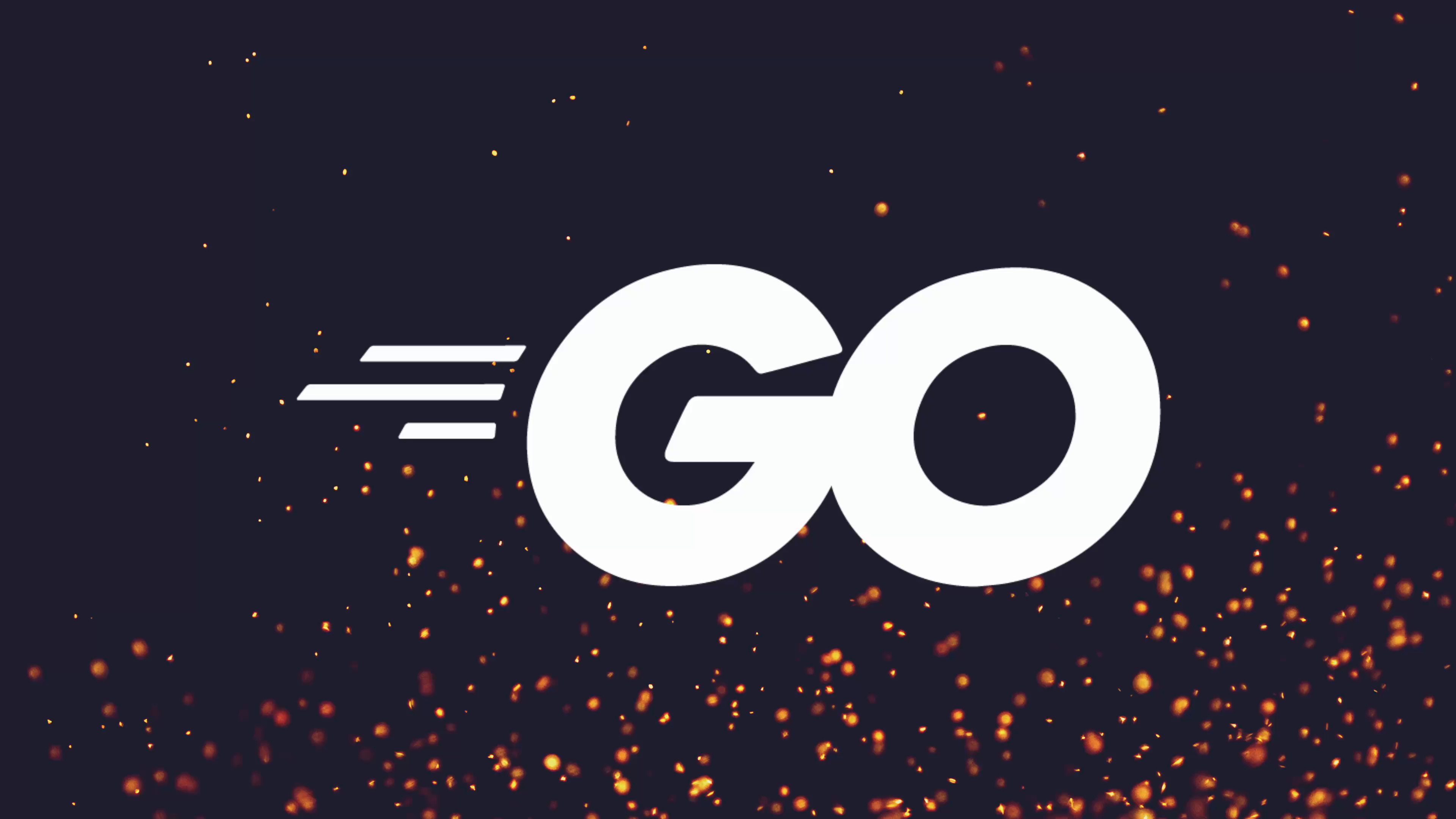
Full Course
In Go, understanding the distinction between value receivers and pointer receivers for methods is crucial, as it directly influences the behavior and performance of functions. While both receiver types can yield the same outcomes in certain scenarios, pointer receivers allow for modification of the original type's values, avoiding the overhead of copying large structs. This lesson demonstrates the implementation of a new add
method for a count struct, illustrating the practical differences between the two approaches. It emphasizes the importance of consistency in receiver types for struct methods to prevent issues when using interfaces, and encourages a preference for immutability where feasible. By navigating through test-driven development and method implementation, the lesson provides insight into effective coding practices in Go, ultimately culminating in the creation of a streamlined method for aggregating counts.
No links available for this lesson.
The last lesson we took a look at adding in a variadic parameter to our Print
method in order to make the interface a little cleaner—allowing us to pass in multiple file names if we wanted to, or pass in no file names in the case of our standard input stream.
In this lesson we're going to look a little bit more at methods by making yet another change.
Value vs Pointer Receivers in Go
When it comes to methods in Go, there are actually two different types of methods depending on the type of receiver:
- Value receiver — which is what we're using
- Pointer receiver — which we can define like so:
func (c *Counts) Add(other Counts)
In the case of our Print
function, it doesn't matter which one we use—it will behave the same either way. But for some methods, the type of receiver can significantly impact behavior.
Adding a Method to Combine Counts
Let’s add a method that allows one Counts
instance to add values from another. The idea:
totals.Add(counts)
To begin, let’s define the method in count.go
:
func (c Counts) Add(other Counts) {
c.Bytes += other.Bytes
c.Words += other.Words
c.Lines += other.Lines
}
Before we implement it, let’s follow TDD and write a test first.
Writing the Test
In count_test.go
:
func TestAddCounts(t *testing.T) {
testCases := []struct {
name string
input struct {
counts Counts
other Counts
}
want Counts
}{
{
name: "simple add by one",
input: struct {
counts Counts
other Counts
}{
counts: Counts{Lines: 1, Words: 5, Bytes: 24},
other: Counts{Lines: 1, Words: 1, Bytes: 1},
},
want: Counts{Lines: 2, Words: 6, Bytes: 25},
},
}
for _, tc := range testCases {
t.Run(tc.name, func(t *testing.T) {
totals := tc.input.counts
totals.Add(tc.input.other)
if totals != tc.want {
t.Logf("expected: %v, got: %v", tc.want, totals)
t.Fail()
}
})
}
}
Now run the tests:
go test
You’ll see it fails, even though the logic seems correct.
Why the Test Fails
Because we used a value receiver, the method operates on a copy of the struct. It does not mutate the original struct passed in.
We need a pointer receiver to mutate the original struct:
func (c *Counts) Add(other Counts) {
c.Bytes += other.Bytes
c.Words += other.Words
c.Lines += other.Lines
}
Now if you rerun the test:
go test
✅ It passes!
When to Use Pointer Receivers
From the Go documentation:
There are two reasons to use a pointer receiver:
- To modify the value the receiver points to
- To avoid copying the value on each method call (useful for large structs)
Mixing Pointer and Value Receivers
Go discourages mixing receiver types on the same struct. Our code now has:
Print(filenames ...string)
— value receiverAdd(other Counts)
— pointer receiver
This creates problems with interfaces.
For example, if we declare:
type Adder interface {
Add(Counts)
}
Then:
*Counts
implementsAdder
Counts
does not
Because Go treats these as different types.
Preferred Alternative: Pure Value Receiver
Let’s rewrite Add
as a pure value receiver that returns a new Counts
:
func (c Counts) Add(other Counts) Counts {
return Counts{
Bytes: c.Bytes + other.Bytes,
Words: c.Words + other.Words,
Lines: c.Lines + other.Lines,
}
}
Update the test:
totals := tc.input.counts.Add(tc.input.other)
And in main.go
:
totals = totals.Add(counts)
This is cleaner, avoids mutation, and keeps our API consistent.
Summary
- ✅ We explored pointer vs value receivers
- ✅ Created a new
Add
method forCounts
- ✅ Used TDD to verify behavior
- ✅ Avoided mixing pointer/value receivers for interface safety
- ✅ Improved immutability and readability
Now’s a great time to commit:
git add main.go count.go count_test.go
git commit -m "feat: add Add method and refactor with value receiver"
In the next lesson, we'll implement CLI flags to toggle display behavior.
See you there.