Please purchase the course to watch this video.
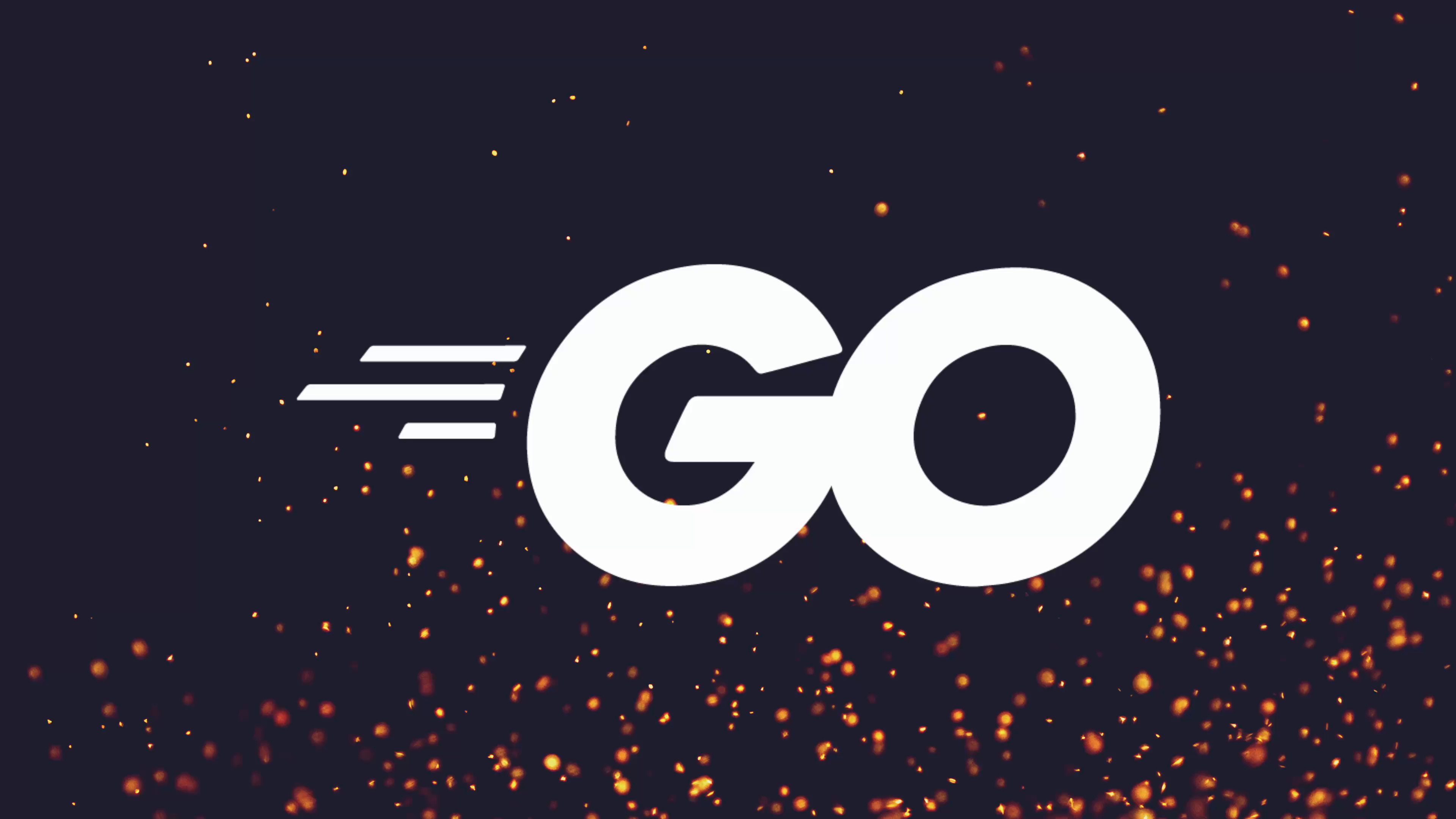
Full Course
Effective project structure is crucial for developing maintainable and reusable code in Go. A well-organized codebase enhances clarity and allows for easier sharing of packages. The lesson emphasizes the importance of separating functionality into packages, such as creating dedicated counter
and display
directories, to avoid cluttering the main package. This modular approach not only facilitates code reuse across different projects but also supports better testing practices by utilizing public interfaces. Additionally, it highlights common practices, such as employing a cmd
directory for the main application logic, which simplifies imports and improves the developer experience. Ultimately, understanding the flexibility in structuring code empowers developers to tailor their approach to fit their specific needs while maintaining good practices for future collaboration and scalability.
No links available for this lesson.
With most of the application's features now complete, in this lesson we're going to take a moment to quickly talk about how we can structure our projects, or the various options we have when it comes to doing so.
Understanding main
Package Requirements
Currently, all of our code is sitting within the root of our project directory inside the main
package. This is required for executable applications.
If we try to rename the package to something else, like counter
, and then run the code, we’ll get the following error:
package github.com/dreamsofcode-io/counter is not a main package.
We must ensure that our application's entry point resides in the main
package.
Creating a Reusable counter
Package
To better separate concerns, we can move our counting logic into its own package.
-
Create a directory called
counter
-
Move
count.go
andcount_test.go
intocounter/
-
Update package declarations:
// count.go package counter // count_test.go package counter_test
-
Update imports in
main.go
:import "github.com/dreamsofcode-io/counter/counter"
-
Move any shared types (like
DisplayOptions
) intocounter/display.go
and update them to be exported.
Running Tests and Application
Once your logic has been moved into the counter
package, you can run tests:
go test ./counter
And run the app as before:
go run . words.txt
Creating a display
Package
To avoid mixing display logic with business logic, extract display code into its own package:
- Create
display/display.go
- Move display-related functions and
DisplayOptions
there - Update references in other files:
import "github.com/dreamsofcode-io/counter/display" var ops = display.Options{...}
Directory Structure
At this point your project might look like:
/counter
count.go
count_test.go
/display
display.go
main.go
The main
function now simply handles CLI parsing and delegates to the other packages.
Reusing the counter
Package
You can now import counter
into other projects:
import "github.com/dreamsofcode-io/counter/counter"
If the package isn't published, use a replace
directive in your go.mod
:
replace github.com/dreamsofcode-io/counter => ../path/to/local/counter
Run:
go mod tidy
Now you can use it in code like this:
r := strings.NewReader("hello world 123")
words := counter.CountWords(r)
fmt.Println(words) // Output: 3
This works because CountWords
is an exported function.
Avoiding Redundant Imports
If you notice your import path looks like this:
github.com/dreamsofcode-io/counter/counter
you can restructure the code to avoid this repetition by promoting counter
package code to the root directory, and placing the CLI entry point in a cmd
subdirectory.
Example:
- Move CLI entry point to
cmd/counter/main.go
- Move business logic to the root (
counter/
package) - Fix imports accordingly
- Build using:
cd cmd/counter
go build -o counter
./counter words.txt
Benefits of This Structure
cmd/
is used for CLI entry points- Root-level packages (like
counter
,display
) are importable by other projects - Clean separation of responsibilities
Committing the Restructure
To save the restructure:
git checkout -b project-restructure
git add .
git commit -m "added in a slight restructuring"
Coming Up Next
In the next lesson, we'll look at package visibility and how it helps in organizing and protecting your code.