Please purchase the course to watch this video.
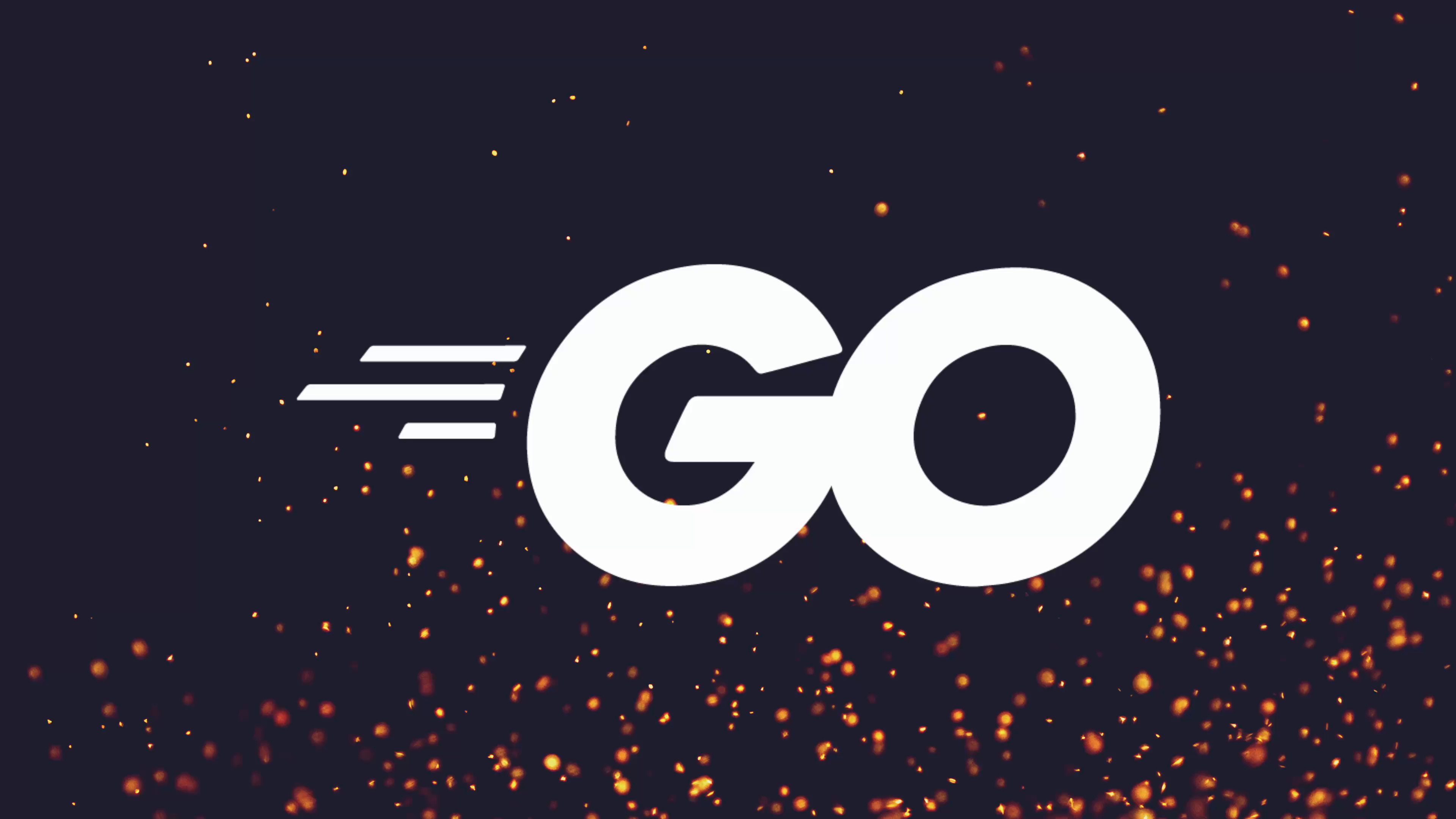
Full Course
The implementation of a count_lines
function in Go enhances functionality by allowing developers to count the number of newline characters within an io.Reader
, a common task when processing text files. Utilizing a Test-Driven Development (TDD) approach, a series of test cases are created first, including scenarios for simple inputs, edge cases like empty files, and inputs without trailing newlines. Initially, a bufio.Scanner
is employed, but it proves inadequate due to its inherent behavior of counting lines regardless of newlines. Thus, the solution shifts to using a bufio.Reader
, reading individual runes to accurately tally the newline characters. This adaptive methodology not only reinforces the reliability of the implementation but also makes it clear how effective testing can guide development and ensure functionality aligns with intended outcomes.
No links available for this lesson.
With our code now refactored into the new count.go
file, let's go ahead and begin adding in our first new counting feature, which is going to be the ability to count the number of lines inside of an io.Reader
.
To do so, let's begin by first creating a new function called CountLines
, which will take a reader or an io.Reader
as a parameter, and again will return an integer representing the number of new lines in our file. Then for the moment, let's go ahead and return the value of 0
.
Before we add any code into this function, in order to start counting the number of new lines inside of an io.Reader
, let's take the same approach we did when it came to our CountWords
function, and use a TDD approach in order to prove that our code is correct. To do so, let's head on over to our count_test.go
function, which contains our test CountWords
test cases that we already have set up.
Here, let's go ahead and add in our new function in order to test the CountLines
function, taking in a testing.T
as the parameter. The easiest way for us to have multiple test cases and to add new ones is to use parametric testing through the use of table driven tests. So let's go ahead and define our test cases as such, which is going to be a slice of anonymous struct, which will contain a name
, which is going to be a string, an input
, which again is going to be a string, and an expectation
, or a wants
, which again is going to be an integer. Then we'll set this to be an empty slice as follows.
Next, we then want to iterate over these test cases: range
of test cases, and we'll call a new subtest on each one. So tc.name
function of t
. And then inside of this, we can go ahead and implement our actual test code, which is to first create an io.Reader
, or a strings.Reader
, which we can do as r = strings.NewReader
created from the tc.input
. Then we can go ahead and pass this reader into the counter.CountLines
function as r
, and let's go ahead and capture the return value in a variable called numLine
.
Next, we can then go ahead and assert that the number of lines is correct, which we can do by doing the following: if numLines is not equal to tc.once
. Then we'll do a t.Logf
, do the format log of expected %d, got %d
. The %d
in this format string is used to represent a digit or an integer. In this case, we want to do expected tc.once
, and we actually got the number of lines, or the result. I'm going to actually change this to be res
because it's a little easier to understand that this is the result. And we'll do a t.Fail
, letting the test know something went wrong.
With that, we have our basic test function and code defined. Now let's go about adding in some test cases. For myself, I always like to begin with a happy path test, which is basically to add a really simple test case and to be sure that it passes. So we'll go ahead and say "simple five words, one new line". If you'll remember, we're counting the number of new lines in the file, not the number of lines, which has a slight bit of difference, which we'll see when we go to implement our algorithm. In a case, this is going to be an input of one, two, three, four, five
, followed by adding in a new line character at the end. The \n
, when it comes to Unix systems, represents a new line character. Here, we want to have the result of 1
. With that, we have our first test case added in.
As I mentioned at the start, we're going to take a more TDD approach when it comes to this implementation. Which means, rather than adding in more test expectations, we're just going to write code to make this test pass, before refactoring it later on. Therefore in order to do so, let's first validate that this code is actually failing, which we can do running the go test
command as follows.
As you can see, our test counts, simple five words, one new line, fails. As we expected 1
, but we got the result back of 0
. Therefore let's head on back over to our CountLines
function and fix this to work. The simplest way to solve this is going to be to take a very similar approach to our CountWords
function. Which is where we're going to use a bufio.Scanner
. By default, the new scanner will split on lines, rather than on words. Meaning that our CountLines
function can be very similar to our CountWords
function. Except rather than splitting on ScanWords
, we can actually just omit this. So let's go ahead and do the same thing. So let's go ahead and define a variable called linesCount
, which we'll set to be 0
. And we'll make sure to return it as well.
Then we're going to define a scanner, which is a bufio.NewScanner
, which will take our io.Reader
as an input, which in this case we've defined as r
. As you can see, by default, the new scanner function returns a new scanner to read from r
, and the split function defaults to ScanLines
. So we don't need to define the split function ourselves. Then we can use the for scanner.Scan()
method, linesCount++
. If we go ahead and save this file and head on over to a terminal window, followed by running the go test
command as follows, you can now see our tests are passing. And we've sufficiently managed to solve our first test case. Great.
Let's go ahead and add in another. This one is going to be an edge case, which is going to be an empty file. Keep this all lowercase just to be safe. Then for the input of this file, let's go ahead and set it to be empty. In this case, we want to have an, in this case, our expectation or our wants should be 0
. By the way, I'm just going to go ahead and lowercase these because we should have them as lower cased. That's something I've just made a mistake on, so going to go ahead and do this as follows.
Okay. Oh, yeah. We need to make the same changes here. That's better. You can have either uppercase or lowercase names here. For me personally, it's much clearer to use a lowercase name just because it communicates to any future readers that we're not exporting this type, which we're not. In any case, now that we have our second test case, which is of empty file and the expectation or wants is 0
. Now if we go ahead and test this code, you can see it works. Great.
Let's go ahead and add in yet another test case. This one should be no new lines. In this case, we're going to have an input of one, two, three, four, five
, but there'll be no new line at the end of the file. In this case, our expectation again should be 0
. Remember we're counting the number of new lines, which is the same as the wc
command does. Print newline count. To show you what this looks like, if I go ahead and use the wc
command as follows, counting the number of lines, and if I type in one, two, three, four, five
, and just press ctrl+d
, you'll see the count is 0
, although it gets printed on the same line. Therefore in this case, we're expecting our count here to be 0
as well.
However, when I go and test this code, you can see it fails. Instead, we get back the value of 1
. This is because the bufio.NewScanner
is still returning the first line, even though it doesn't have a new line in it. Additionally, if we go ahead and add in yet another test case, well, this time we'll do no new line at end, we'll do a \n
, we'll do six
and leave it as so. Here we're expecting there to be the value of 1
. But when we go and test this, you'll see it comes back with the value of 2
.
We could try changing this by just removing the number of lines by one. However, when I go ahead and do this, you'll see that the test cases of simple five words and empty file are now returning the incorrect results. This means that this isn't a solution to the problem we currently have.
So how do we go about solving this problem? Well, unfortunately, the issue is, the new scanner just won't work for our use case, as it will always return a line whether or not it has a new line in it. It just splits the code up depending on how many new lines there are. So instead, we're going to need to take a different approach. One that was actually similar to what we first implemented in the CountWords
function when we began looking at the bufio
package, which was to use a reader
and check the individual runes to see if they were a space character.
Therefore, to do so, let's first get rid of the code where we're scanning through individual lines, and instead go ahead and instantiate a new bufio.Reader
using the NewReader
function, passing in our reader. Now, with our reader defined, let's go ahead and implement a similar algorithm to what we saw before. We're starting with adding in a for
loop so we can iterate over each of the individual runes. Then we can access the rune, assigning it to a variable as r
, we'll ignore the size value for the moment, and capture the error as well from the reader.ReadRune
method, which as you can see returns the rune, size and error.
If we have an error, which we can check using the if err is not equal to nil
, we just want to break out of this loop. This error will occur at the end of a file or if something goes wrong with our actual ReadRune
method. Either case, we want to exit out of the loop and just return the line count. Next, we then need to go ahead and check the value of our rune. So if r is equal to \n
, then we can go ahead and just increment the line count by 1
, which we can do using lineCounts++
. With that, our algorithm should be good to go.
If we go ahead and test this code using the go test
command, you can see it now passes for all of our line test cases. If we head back on over to the count_test.go
function, let's go ahead and make sure that everything is working correctly by adding in a couple of multiline strings, multi newline string inputs. Here we're just going to do \n, \n, \n, \n
, which should have the value of 4
, that's 4
new lines. And let's go ahead and add in another multiline string. And in this case, I'm going to go ahead and do 1 \n 2 \n 3 \n 4 \n 5 \n
. And this should have the value of 5
.
Now if we go ahead and run the go test
command to test our new test cases, again, we can see that they're all working, which gives us the confidence that our code is working as expected. With that, we have the new CountLines
function ready to go. The next thing we want to do is add in our CountBytes
function before making the necessary changes to our CountWordsInFile
function to include them as results.
Before we move on, go ahead and add in the count.go
and the count_test.go
files into your git repository and commit it with a similar commit message to mine, added new CountLines
function. With that, I'll then see you on the next lesson.