Please purchase the course to watch this video.
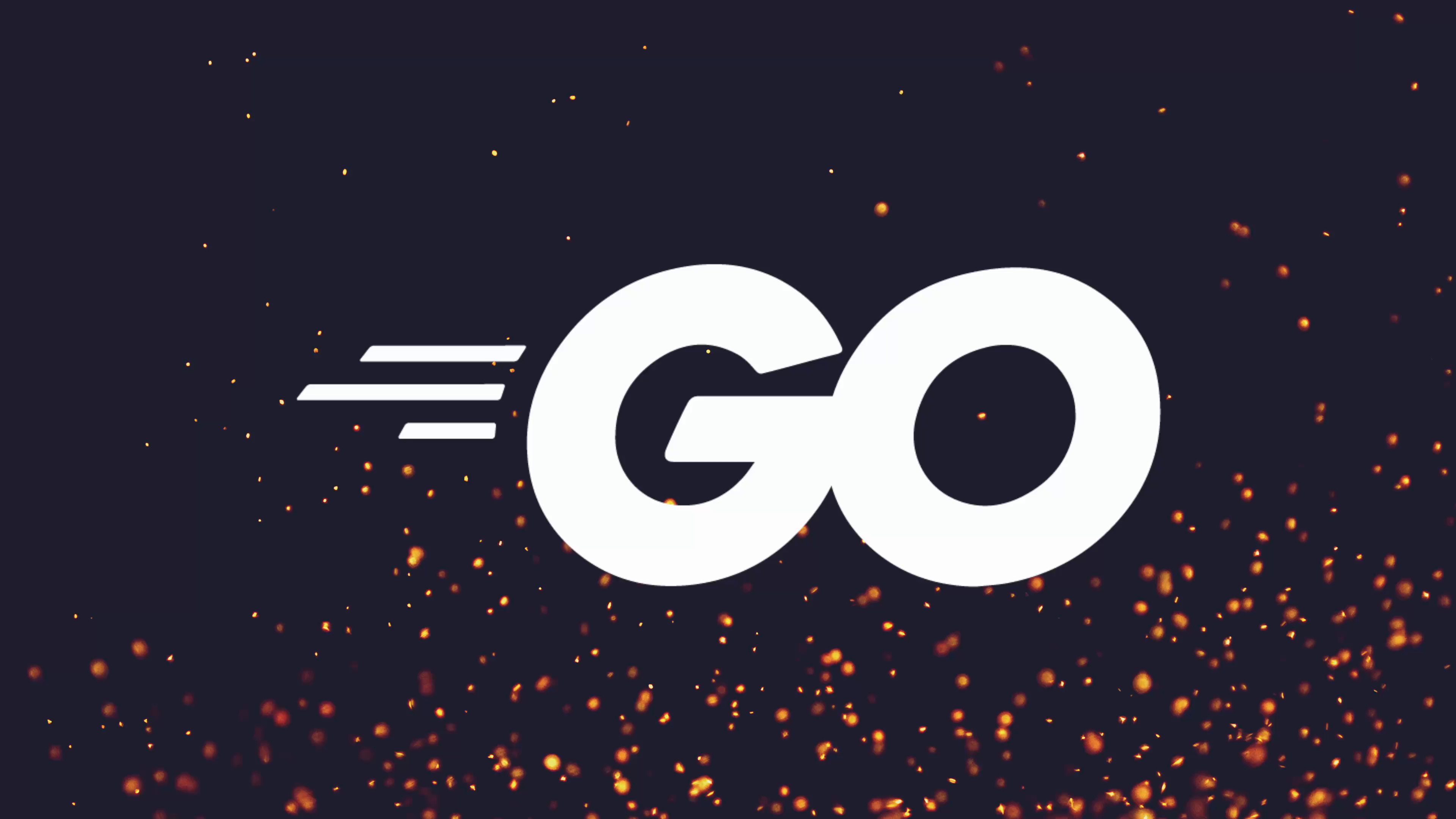
Full Course
Working with files in Go involves understanding how to effectively write data while managing file modes and permissions. Unlike reading files, writing data can present challenges, particularly regarding whether to append to an existing file or truncate it. The os.create
function can be convenient but will replace existing content unless specified otherwise. To append data instead, the os.openFile
function is preferred as it provides more control over file behavior, including flags for read and write permissions and the ability to use O_APPEND
to add data without losing existing content. Correctly setting permissions, such as 0644
, governs user access to the file and ensures that proper read/write privileges are enforced. Understanding these aspects not only simplifies code management but also enhances data integrity when building CLI applications.
No links available for this lesson.
Throughout this course, we've done quite a bit of work when it comes to files. However, for the most part, it's mainly been reading data from them rather than writing data to them. At this point, you may feel like writing data to a file is pretty easy, or especially considering that the os.File
type conforms to the io.Writer
interface, which is something we've used often throughout this course. However, when it comes to working with files in the operating system through a CLI application, there's actually a lot more we need to consider, specifically when it comes to file modes and permissions, each of which will differ depending on what our intent is.
In order to show the various situations that we might encounter and how we can modify the way that we interact with files on the operating system, let's begin by just writing some arbitrary data to a file. To do so, I'm going to go ahead and create a new file called data
, using the create
function of the os
package. Let's go ahead and capture these return values as well. We'll also go ahead and add in a log.Fatal
line, just so that we can see what goes on when we change some of the ways that we create a file in a minute. Failed to create file. Let's go ahead and add this error to the log.Fatal
line.
Now, with our file created, let's go ahead and write some data to it. In my case, I'm going to go ahead and use the Fprintln
method of the fmt
package, passing in our file as the io.Writer
, and then writing the following line. To make this a bit easier, I'm just going to go ahead and use the os.Args
and pass in the first value. Lastly, all that remains is to make sure we close this file at the end of our function, although this will be automatically closed by the operating system in case anything goes wrong. So this line isn't exactly needed for a CLI application, but it's a good habit to close your files whenever you open them.
Now, if we go ahead and open up a new terminal window and do a quick ls
, we can see that there's no file created. Let's go ahead and run this code using the go run
command, and we'll pass in the string that we want to be written to our data file. Let's go ahead and pass in hello world
, and we'll need to escape the world
. Now, if we take a look, you can see we have a data
file written to our directory, and if I cat
it, you can see it contains our hello world
string. Pretty simple.
However, if I go ahead and run this code again, passing in a different line of foo bar
, now when I go ahead and cat
the data again, you can see that the original line we have written of hello world
no longer exists, and we only have a single line of foo bar
. So what is actually going on? If we take a look at the documentation for the create
function of the os
package, you can see it creates or truncates the named file. This means if the file doesn't exist, it creates it, and if the file already exists, it is truncated. In computer science, when a file is truncated, all of the data is cut off or removed, and therefore when we create a new file, we overwrite all of the existing data.
If we take a look at this documentation a little more, you'll also notice that it says if the file does not exist, it is created with the mode octal 666 before you mask. If successful, then methods on the file can be used for IO, and the associated file descriptor has the mode of O_RDWR
. And if there's an error, it will be of type path.Error
. So as you can see, there's a lot of things that are happening inside of the create
function that we haven't specifically defined.
Basically, the create
function has a number of defaults when it comes to creating or opening a file. If the situation you're in works with these defaults, then by all means go ahead and use this function. However, let's say in our case we don't want to truncate the file, but we want to append to it. How do we do so? Well, in Go we need to use another function, which is the OpenFile
function of the os
package, rather than the create
function instead. If we take a look at the documentation for this, you can see that it's the generalized open call, rather than a specialized open call, which the create
function was, and also the open
function is as well.
The OpenFile
function opens a named file for reading, using the read-only mode. In our case, we want to write to this file, but we want to ensure that we're only appending data, rather than truncating it. As you can see, the OpenFile
function takes three parameters. The first is the name of the file that we want to open, or create if it doesn't exist and we've specified the O_CREATE
flag. More on that in a minute.
The second parameter is the list of flags, such as O_RDONLY
, O_CREATE
, that we can use in order to specify the behavior of creating or truncating or appending to this file. Again, we'll take a look at that shortly. The third option are the permissions, or the file mode. This parameter is used if the file doesn't exist, and the O_CREATE
flag is passed in. Then it will be created with the mode perm
. The documentation also provides a couple of examples on what you can use the OpenFile
with.
In this first example, they're opening a notes.txt
with read-write permissions, and a create permissions if the file doesn't exist, and it sets the permissions to be 0644
. We'll take a look at what those permissions are later on, and how we can read them, but for the meantime, this just means that the current user can read and write, the user's group can only read, and all other users can only read as well.
The second example is actually what we want to implement with our code, which is opening an access log and appending to it, as well as creating and specifying the read-write mode to be write-only, sorry. Again, it's creating it with the same permissions we saw before, which allows the current user to write and everyone else to read. With that, let's go ahead and change our code to make use of the OpenFile
function instead, allowing us to create the file if it doesn't exist, and append to it if there's data already.
To do so, we can use the OpenFile
function, as we've seen before, passing in the name of the file we want, called data
. In this case, I'm going to call it data.txt
, so we have a new file. Next, we then need to pass in our file modes. You can find a list of all of the different flags that we can pass into the file under the os
package's documentation, under constants.
Here you can see we have three flags that exactly one of them must be specified when it comes to our files. These are read-only, for files that we only want to read from, write-only, for files that we only want to write to, and read-write files, for files that we want to both read from and write to at the same time. Specifying the correct permission for your use case is really important, as it can constrain the amount of behavior that you have for a file.
In our case, we only want to write to this file instead of reading from it, so let's go ahead and pass in the os.O_WRONLY
flag. Next, we then want to add in some remaining values in order to control the behavior. These are added using a bitwise or, which we'll take a look at in a minute. In our case, we want to append data to the file when writing, rather than truncating it. So we can go ahead and or
this in using the os.O_APPEND
flag. This means if the file exists, we'll append to it instead of truncating it. Pretty cool.
Let's go ahead and leave this as follows, and add in the following permissions. For the moment, let's go ahead and just set this to be 0644
, which is the same that we saw in the existing examples. We'll talk a little bit more about file permissions in the next video. However, for the moment, you can understand the first number to be the files owner, and in this case, it's set to read write permissions. The second number is the files user. In this case, it's set to read permissions.
And the third is known as other, which is everybody other than the files user or the file or any members of the files group. And in this case, this is set to read as well. Now, if we go ahead and run this code using the go run
command, passing in the string we want to write, you'll see we actually get an error. Although this is a bad error message. Let me go ahead and change this again. Failed to open file. Now let's go ahead and run this code again, just so that we can see the error a little bit more clearly.
So the error we get back is failed to open file. Open data.txt, no such file or directory. This error is correct. We don't have a data.txt
file within our code. However, if I go ahead and change this to be just data
without the txt
extension, and I run the command again using this is line two, you'll see that this now passes. And you can see we've appended our string to it.
So why is this failing when it comes to data.txt
? Well, this is because we haven't set the correct mode in order to create the file if it doesn't exist. To do so, we need to add in the O_CREATE
option as follows using os.O_CREATE
. This option will create the file if it doesn't exist. Being able to set or omit this flag is pretty useful for certain situations, such as being able to open and write to a file if it exists already, or as we saw, constraining our open file operation to only creating a file if it doesn't already exist.
Let's go ahead and test this out by running the code again, saying writing to our new file. As you can see, we didn't get an error and the data.txt
file exists. And if I go ahead and print the contents of it, you can see we have our new line. Let's go ahead and do something else, such as line number two. And you can see data.txt
, there it is. By using the OpenFile
function and setting the different behaviors we want, we're able to easily constrain the different scenarios that we may want when it comes to opening and writing to files.
As I mentioned before, you can see all of the different modes inside of the constants of the os
package, which will allow you to either append, create, exclusively create, which means O_CREATE
the file must not exist, open up for synchronous IO, which is something you may want to consider, or sending a flag to truncate the data in the file if it already exists. One last flag that I think is worth talking about is the O_EXCLUSIVE
.
This mode forces the file. This mode tells the operating system to only create a file if it doesn't exist already, and will cause an error if the file already exists. For example, let's go ahead and remove the data file and use the go run
command to create a new file, which as you can see works as expected, and also has the bit mask flag of 600
. However, if I go to run my code again, you can see this time it actually fails. This is because the file already exists. This is useful when it comes to things such as file locking, where say you only want the application to run if a file doesn't exist already, and should help to clarify when to use the more specialized functions of os.Open
and os.Create
, as well as when you may want to create your own specializations using the OpenFile
function.
In any case, that covers the basics of file modes, and how you can use them to constrain the capabilities of your file operations, allowing you to do things such as creating a file if it doesn't exist, appending to a file's existing contents when you write to it, or just truncating the file entirely. In the next lesson, we're going to do a deep dive into permissions, specifically taking a look at how we can actually set, specifically taking a look at how permissions work when it comes to a Unix system, and how we can go about setting them both on the terminal, and within our Go code as well, when we both open a file, and if we want to modify the permissions of a file that already exists.