Please purchase the course to watch this video.
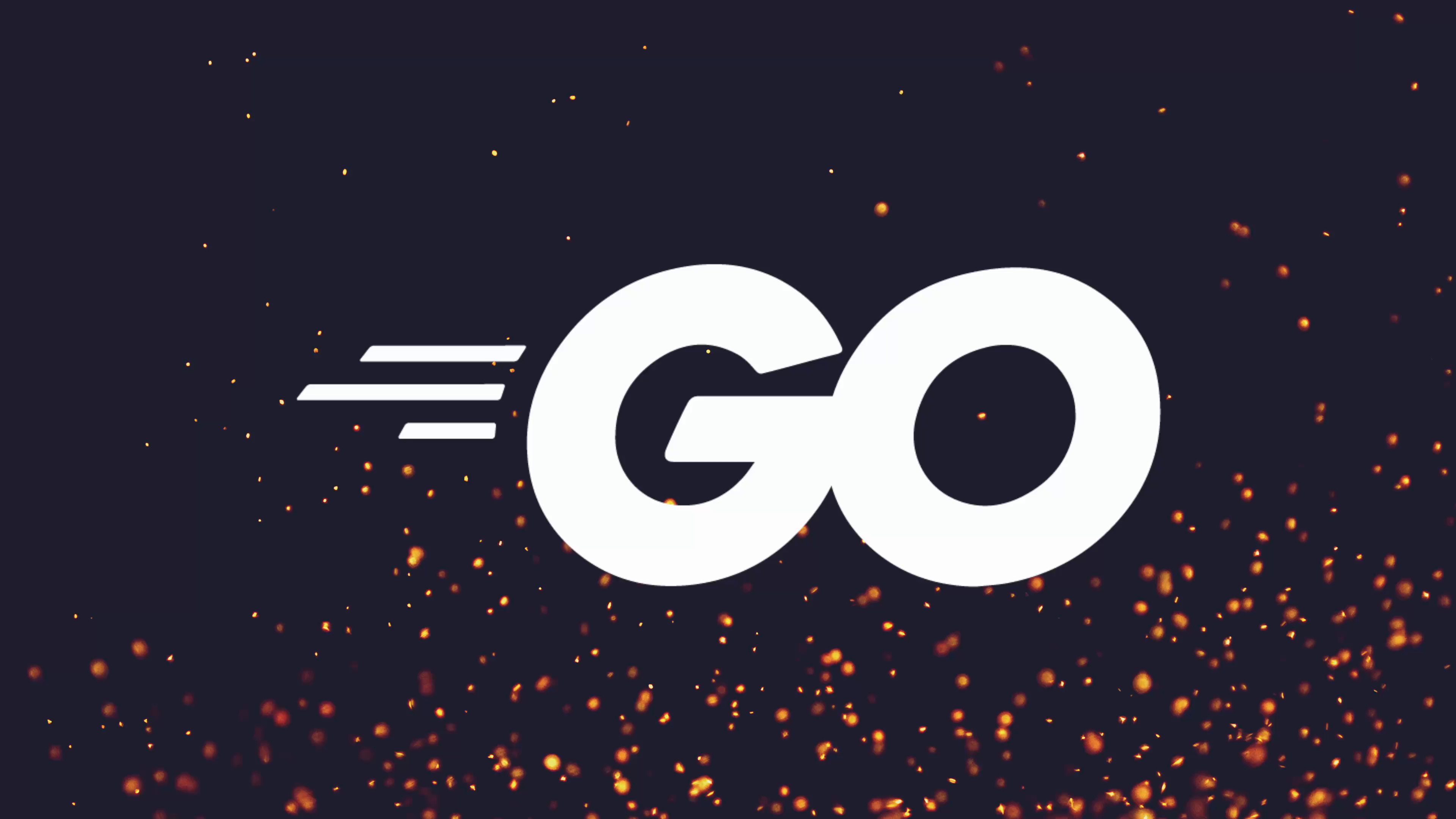
Full Course
Standard input is a powerful feature in command-line interfaces that allows data to be passed directly into applications, enhancing their functionality and flexibility. By integrating standard input, applications can accept user input dynamically, functioning similarly to file inputs. This capability is particularly useful for chaining commands through the pipe operator, enabling seamless data flow between processes. For instance, leveraging standard input in a word counter application allows for both file-based and direct user inputs, providing versatility in how the application can be used. Implementing this stream not only simplifies user interactions but also strengthens the application's design by allowing it to handle various input methods efficiently. As developers build CLI applications, understanding and utilizing standard input can significantly enhance the user experience and the overall robustness of the application.
No links available for this lesson.
In this lesson, we're going to look at the third of the three data streams that every process has available to it, the standard input stream, and how we can make use of it in our own CLI application to make it even more versatile. If you'll remember, the two other data streams that an application has available to it are standard out and standard error, which are both used for communicating messages to other processes or to our users via the console.
The standard input stream, however, is slightly different, as it allows data to be passed into the application, meaning you can use it in place of a file. This makes it extremely powerful when it comes to things such as chaining commands using the pipe operator, which we'll take a look at in a minute.
If we head on over to our word counter application, currently we're able to specify the file or files we want to pass in through command line arguments, such as follows. In this case, we're counting the instructions that are found in the words.txt
and lines.txt
files. If we go ahead and take a look at the wc
command that we've been using as a reference for our own application, you can see the same thing takes place here as well, where we can pass in multiple files and we'll get the word counts for each of those.
However, let's take a look at the behaviour of the wc
command when we don't pass any files in as arguments. As you can see, the application sort of waits. This may feel like it's stuck or hanging, but actually that's not the case. For instance, I can go ahead and type in the following string of hello world one two three
. And if I go ahead and press the ctrl+D
command, you can see it prints out the number 5
.
So what just happened? Well, in this case, we actually just entered input into the wc
command, which was in the form of the input buffer that I typed out. Then, when I pressed the ctrl+D
commands, it caused the input buffer to end, or end of file, which the wc
command then went and counted all of the words that I had entered into. And as you can see, we got back the result of 5
, which correlates with the number of words that we passed in.
The way this works is when the wc
command receives no file names that have been passed in as a command line argument, it then reads from the standard input file instead, which is what we were typing into. Then by pressing ctrl+D
, I caused this file to end of file, or to close. And the wc
command then went and counted the contents within.
Whilst being able to pass in a data stream through standard input is cool in itself, and has some real valuable uses when it comes to things such as password entries, it's not the only thing you can use the stream for. In fact, one of its main uses is actually as a pipe for other commands. For example, if I run the following echo
command of echo foo barb az
, and go ahead and just enter this, as you can see, it prints the words foo bar baz
to standard out, which appears on our console.
However, if I go ahead and run this command again, but this time use the following pipe operator, then passing it into the wc -w
command, this time when I execute this, you can see we get back the number 3
. This is happening because we've passed in the standard output of the echo
command into the standard input of the wc
command, through the use of the pipe operator, which takes the standard out of the previous command, and passes it into the standard input of the command afterwards.
Being able to pipe commands like this is actually a powerful feature of command line interfaces, and why the terminal is so powerful. For instance, you can also chain commands as well, such as being able to do foo bar baz
, piping it into wc -w
, and then we could even pipe that into the word count application again, which obviously would return 1
. But let's say you want to use other commands, such as piping in the {"foo": "bar"}
JSON string to the jq
command, and then we could even pass this to the wc
command as well, which produces the value of 4
.
As I mentioned, the ability to pipe commands is what makes the CLI so powerful. So when it comes to building our own CLI applications, such as our word counter, it's a good idea for us to also support the standard input file stream. So let's go ahead and add it in.
In order to do so, the first thing we're going to want to do is take the standard input stream whenever we don't pass in a file. However, currently, as you can see, our application produces an error if we pass no files in as an argument. Therefore, let's go ahead and remove this validation check on lines 18
to 20
.
Now if I go ahead and run this code, nothing happens, but at least we're no longer getting an error. Next, we then need to add in a check if the length of file names is 0
, then we're going to use the standard input stream. To do so, let's go ahead and do:
if len(fileNames) == 0 {
// handle stdin
}
But how can we get access to the standard in file? Well, similar to standard error, which you can see on line 22
, and standard out, the standard in file is available in the os
package under the Stdin
variable. As you can see, the standard in is also an os.File
, which, if you'll remember, conforms to the io.Reader
interface. Therefore, because we've set up our code in order to support counting words from an io.Reader
, it means it's going to be incredibly easy to integrate.
All we need to do is call the CountWords
function, passing in the os.Stdin
file. Next, let's go ahead and capture this value in a variable called wordCount
, as follows. With our word count being captured, let's then go ahead and print out the results to the console, using the fmt.Println
function. If you'll notice on line 28
, we're printing out both the word count and the associated file name that this count was derived from.
However, in the case of the standard input file stream, we don't have an associated file name. We could go ahead and print out standard in
as follows, but let's go ahead and check to see what the wc
command does before making that decision. In this case, if we go ahead and use the echo
command again, piping it into wc -w
, you can see that we just print out the number of words and no file name or standard in identifier. Therefore, let's go ahead and remove this and leave this as follows.
With that, our code should now be complete. Pretty simple. However, a lot of the work was already done when we moved towards reading in files and the io.Reader
. Again, this is one of the benefits of making sure that you decouple your code from an implementation type so that you can support even more functionality.
In any case, let's go ahead and check to see that our code works as expected. First, by using the go run
command and entering in standard inputs through an input buffer. 1, 2, 3
. Now if I press ctrl+d
, we get back the value of 5
. Perfect. Let's go ahead and make sure it also works with the echo piping command as well. Which we can see it does, getting back the result of 3
.
And just to be sure, let's go ahead and make sure that our code still works by passing in our two files. Which it does. With that, we've managed to add in handling of standard inputs whenever our CLI application doesn't have a file name to work with. As you can probably tell, our little word counter application is starting to feel more and more complete, with a lot of CLI versatility and functionality being built into it.
In the next lesson, we're going to add in yet another input method. However, this time it's going to be through the use of arguments, but a special type called CLI flags. If we take a look at the wc
command, you can actually see one of these CLI flags in the -w
argument that we're parsing in. In any case, before we move on, go ahead and add the changes we just made from the main.go
file into your git repository and commit it as follows. With that, I'll then see you on the next lesson.