Create an account to watch this video
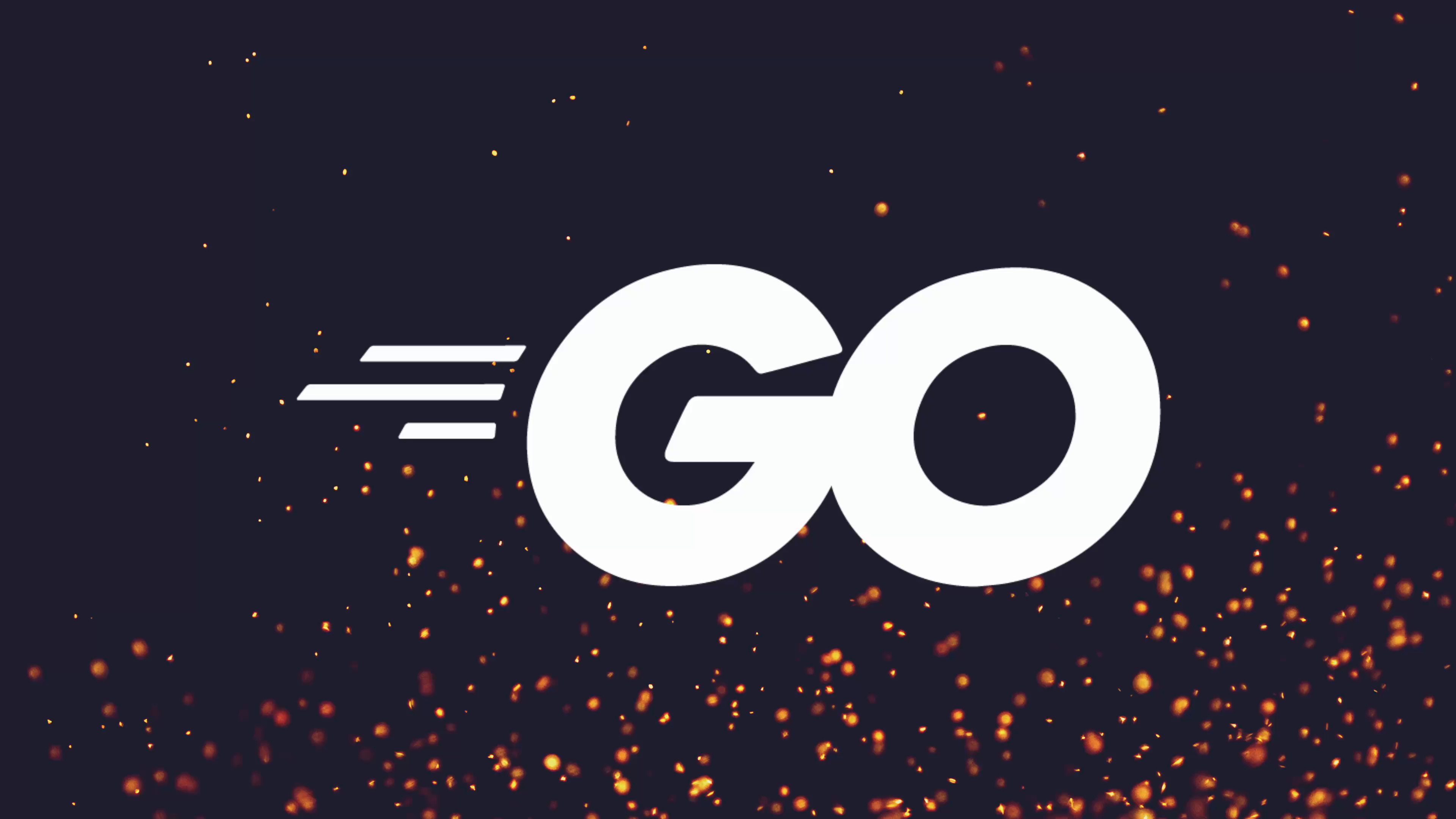
Full Course
Table-driven testing is a powerful technique used in Go to streamline the process of writing and organizing tests, making it easier to handle various cases and expectations without repetitive code. This approach allows developers to define a slice of test cases, each with its parameters and expected results, facilitating concise and clear tests. By implementing named test cases and leveraging subtests, the test output becomes more informative, helping to quickly identify which specific cases fail. Additionally, as the lesson highlights, recognizing the limitations of a simple guard-based approach to input validation prompts an upcoming revision of the algorithm to properly count words, taking into account edge cases such as empty strings, spaces, and newlines to ensure accurate functionality.
In the last lesson, we took a look at adding in some tests for a couple of edge cases that our code wasn't able to handle. The first of these was the empty input, which we did manage to actually solve, by adding in a guard at the start of our code, checking to see if the length of data is equal to zero. However, we then added in another edge case which managed to defeat this guard, which was to add in just a single space, which not only causes our code to fail, but it does so in a way returning the number two instead of returning the number one we had with the empty case.
In this lesson we're going to look at a couple of ways to solve this, but before we do we're going to make it easier for us to be able to add in different testing parameters to this code through a concept called table-driven testing. Table-driven tests are very common when it comes to production Go code, and so they're a really good thing to learn. They're basically a form of parametric testing, which is where you define parameters to test rather than having to write out the boilerplate over and over again.
This blog post by Dave Chaney, which I absolutely recommend reading and I'll leave a link to in the lesson description down below, gives a really good overview of why table-driven tests are great, specifically going beyond 100% coverage. The idea behind them is actually rather simple. For each test case you want, you begin by defining a simple once which is your expectation and any other parameters that you need to pass into your test. Then you iterate over these test cases, or a slice of these test cases, and your boilerplate is only written once. This makes it incredibly simple to go ahead and add all these different input parameters that you want for your test, as well as defining their relevant assertions or expectations, and your code can then work in a really simple way.
So to make our code easier to test, and so that we don't have to keep repeating this boilerplate over and over again, let's go ahead and migrate our current test count words function into a table-driven test. To do so, we first need to define a test case struct, which we're actually going to do inside of our test count words function. This is because we don't need this struct to live outside of this actual function itself, and therefore we're just keeping it scoped to the actual function's case. If we define it outside of this, and then when we start to add other tests later on, this test case struct could conflict with other names. Although later on we're not even going to use a named struct, instead preferring to use an anonymous one. But I'm getting ahead of myself.
In any case, with our test case struct defined, the next thing we want to do is define our actual properties. In this case, all we need is an input, which is of type string, and an expectation, which is going to be our wants. And we'll define this as an int. With our test case struct defined, the next thing we can do is go about defining our individual test cases that we can use to actually test our code. To do so, let's go ahead and define a new variable called testCases
, and we'll set this to be a slice of test case as follows.
Then we can go ahead and define our first test case, which in this case we're just going to go ahead and set to be the exact same code that we have on lines 17 and 18. So our input is going to be one, two, three, four, five
. And our expectation or our wants is going to be five
. And we need to make sure we've got the relevant commas where we want them. Okay, with that, we'll just leave this for the moment as our single test case.
Then it's time to go ahead and actually make use of these with our code. To do so, all we need to do is iterate over each test case using a for range expression. So for tc
, which is going to represent the test case and assigning it to the value of range testCases
, then we can just go ahead and call our code inside. So first defining the results
, which is going to be countWords
, and we'll cast it to a slice of bytes passing in our input string.
Then we can go ahead and perform our single test assertion. In fact, I'm just going to go ahead and copy this code just to make it a little easier, so we don't have to write all this out. And then we just need to change our wants to be tc.wants
, so the wants of our test case. And we need to change this here as well. As you can see, this code is more concise, and not only that, it's really easy for us to be able to define new test cases, as we can just do so as follows.
We'll take a look at that shortly, let's just go ahead and clean up this code for the meantime, and we'll go ahead and make sure that everything works by running the go test
command, which it does. Okay, with that, we can now go about adding in our individual test cases. Let's go ahead and set this one to be an empty string, and we'll define it as zero. As you can see, that's all it takes to add a new test case into this code, much quicker than what we were doing before. Again, let's just go ahead and test that this is working, which it is.
Lastly, let's go ahead and add in the test case for our empty string, which again, we want this to be zero, but we know that this is going to return two. Now, if we go ahead and run this code, as you can see, our code is working as expected. However, there is a bit of an issue with this formatting. If we take a look at this code, you can see we got expected 0 got 2
, and it failed on main_test.go:29
, which is the case.
However, let's say we didn't have our guard in that we added in the last lesson. We'll just go ahead and comment this out for the meantime. If we go ahead and run this code again, using the go test
command, you can see here that we're getting two failures, expected 0 got 1
, expected 0 got 2
, and both are happening on line 29. When looking at this test output, it's very difficult to know which test actually failed. For example, if we head back on over to our main test code and take a look at line 29, whilst we know that this is where the test actually failed, we're not too sure which test it was that failed.
Both of these have an expectation of zero, but one was returning one and the other was returning two, and it's not too clear which one actually was. We can probably make a determination given on the actual ordering. We know that this test occurred before this one. But in some cases, you may want to run these tests in parallel, which makes it even harder to determine which test was the one that actually failed. Fortunately, there is a way to solve this, which if we actually take a look at the documentation, I want to see if Dave mentions. Aha! Give your test cases a name, which is another common pattern.
And then, as you can see, when the test fails, we have a descriptive name for what the test was doing. We no longer have to try to figure it out from the output. So, let's go ahead and actually give our test cases a name. Let's do this with the name of string, and we can then give each test case a simple name. So, in this one, we'll give it five words
. And in this one, we'll give it empty input
. And in this one, let's go ahead and give it single space
. Sorry, not empty. Name. Okay, now that our test cases have a name, how do we go about using them? Because if we just go ahead and run go test
, it doesn't work too well.
Well, we could add the name to the log output. So, we could just start by doing -s
and then putting tc.name
. This is a valid approach, but it's not the best way in my opinion. As you can see, this blog post does mention to use this within the first example, but a better approach in my opinion, and this blog post says as you scroll down, is to introduce the concept of subtests. A subtest, when it comes to our test code, is the ability to run a test inside of this test. Yes, there is that meme going on at the moment.
In order to invoke a subtest, you can use the Run
method of the t
type, which runs f
as a subtest of a t
called name. This name value is what we can pass in from our individual test case, and will allow us to identify the individual test that we're running. There are some other benefits to actually using subtests as well, given that it runs in a separate Go routine, so if there's a panic or a fail now, then this won't actually cause the entire test suite to fail, just that individual test, and not only that, it also means we can run these in parallel if we want to.
Therefore, let's go ahead and make use of them, calling the Run
method and passing in the tc.name
as the first parameter. Then for the second parameter, we need to go ahead and pass in a test function, which has the exact same signature that we are already using, and we can then just go ahead and copy this and paste it in. And we'll go ahead and format it as well, and we'll also get rid of the line on 37. Okay, with that we now have our code running inside of a subtest.
And let's also go ahead and get rid of the actual name output that we added in before. Okay, now if we go ahead and run this code, you should see what it looks like when our test fails. Here you can see each failure is now marked on its own individual line, nested inside of our actual testCountWords
function. And you can see we get the fail testCountWords
, which is the parent function, but our actual test name is being printed out as well. So in this case, empty input
and single space
. This makes it a lot easier for us to actually see where the failure was occurring from.
In this case, empty input
was failing, and in this case single space
. So as you can see, by making use of a subtest, we've managed to make the output of our table driven tests more readable. Okay, with that we now have our parametric testing set up. The last thing I want to do however is to actually simplify this code a little more, which is to move away from using a named type or struct into an anonymous struct. To do so is actually rather simple. Instead of defining this as a slice of test case, we can actually define this as a slice of struct
as follows.
Then we can just define the properties within. So name
is string, input
is string, and our wants
is an int. And with that our code is now working the exact same way as it was before, but we're just omitting the fact that we're naming a struct. This has no actual technical benefits or technical drawback, it just makes the code a little bit more readable, in my opinion. And it tends to be idiomatic when it comes to production Go.
Okay, with that we now have our test cases working. Let's go ahead and add back in our guard as we saw before. And we can now go about fixing this other edge case we have, which is where we're expecting zero but we got two. In order to solve this we're going to need to figure out a way to check to see whether our code has anything more than a non-space character. And if it does, then we want to return our word count, otherwise we can just return zero.
To solve this we need to think of an approach that we can take in order to detect whether or not we had a non-space character. And if we didn't have a space character, only then do we want to take the value and actually return it. In order to do so we can just simply make use of a boolean flag. So let's say we can go ahead and say wordDetected
, and we'll set this to be false initially. Then after we've performed our loop, if we didn't detect a word, wordDetected
, then we'll just go ahead and return zero.
So we're adding in another guard, this time after our actual word iteration. Then in order to set the wordDetected
to be false, we can just go ahead and do the following else expression. So if x
is not a space, we can say wordDetected = true
. With that, now our code should be working. If we go ahead and test it, as you can see it's now passing.
Unfortunately however, adding guards for each of the problems that we come across isn't exactly the most scalable solution when it comes to writing a robust algorithm. For example, if I head on over to the main
test function, let's go ahead and add in another test case that we're currently not handling, which is new lines. For example, let's go ahead and add in the input of one, two, three
, and we'll add in a new line character, which we can do using a backslash n
when it comes to a single line string, or in Go we can actually do so by using a raw string as follows, which will then respect any new lines we pass in.
So four, five
and we'll go ahead and set this to be as follows. So this will have one, two, three
, and then a new line between four
and five
. Either way is going to work, for me I like to keep things on a single line, so I'm going to use the new line character as follows, just to make it a little bit easier for us to see. Okay, for the expectation here we want to get the number five, but if we go ahead and run our tests we can see that we actually get the number four.
This is because we're not treating the backslash n
or the new line character as a space, so it's not tallying up the number of words that we have. Additionally, this isn't the only issue we have. In some cases you may have a sentence that has multiple spaces in between, so let's go ahead and do multiple spaces. For example, in this case you may have this is a sentence
, let's say, and a full stop. And in some forms of writing it's actually customary to have a double space after a full stop or a period, so we can say this is another
.
Whether or not you agree with that, it does happen in the real world and therefore we have an expectation that we should have seven words, but again we're going to have eight. So let's go ahead and test this. As you can see we expected seven but we got eight. We could of course go ahead and write code in order to solve each of these individual test cases, but ultimately we just have to accept that our algorithm isn't fit for purpose.
This is because we're counting the number of spaces instead of actually counting the number of words. Therefore in the next lesson we're going to go ahead and revamp our algorithm in order to make it better fit for our intended use rather than counting the number of spaces instead counting the number of words.