Please purchase the course to watch this video.
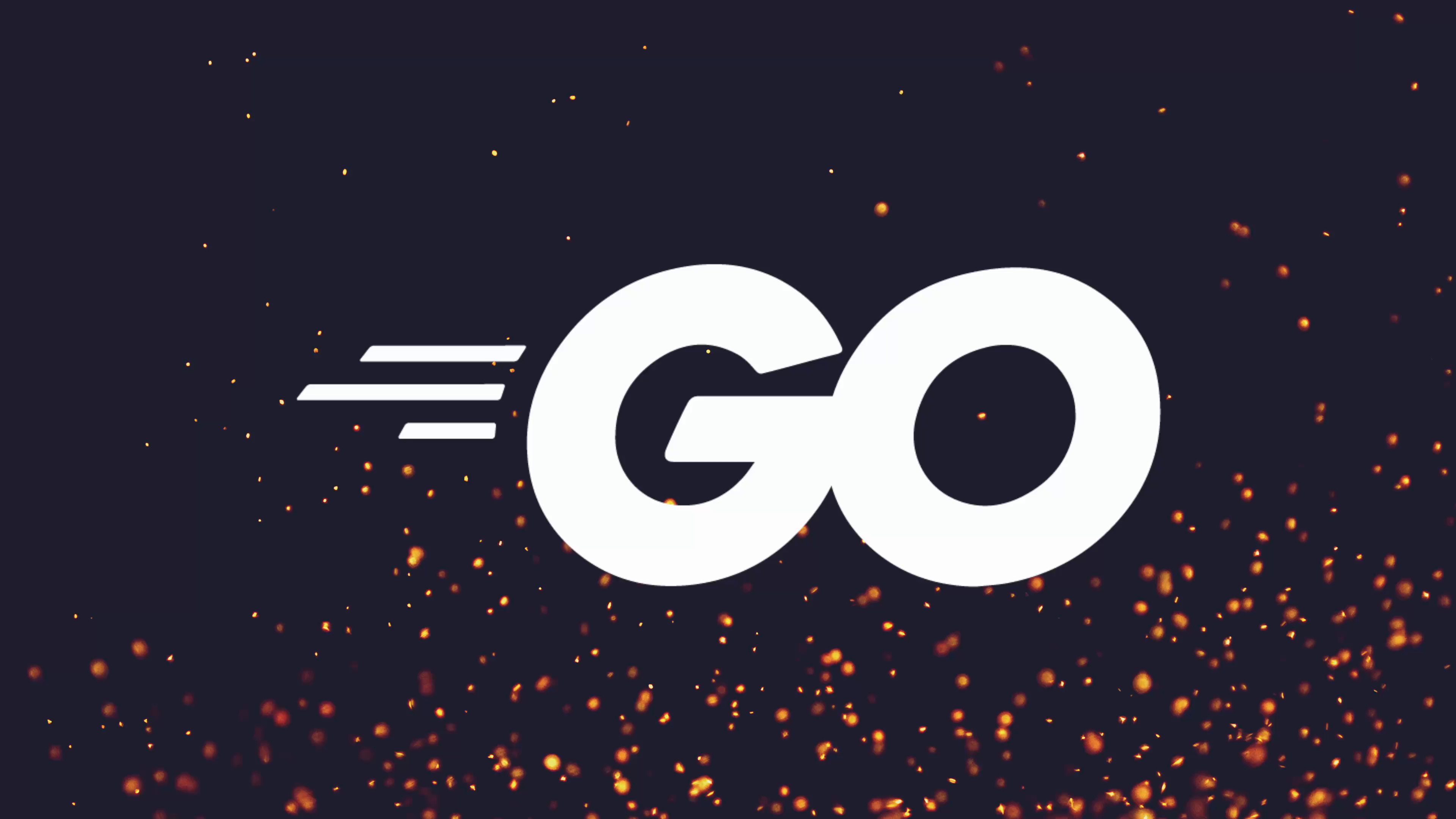
Full Course
Creating effective test assertions is essential for streamlining the testing process in Go, as many assertions tend to follow similar patterns of checking value equality and reporting discrepancies. Given the repetitive nature of these assertions, developing a test helper can significantly enhance code clarity and maintainability. Two approaches to implementing test assertions are discussed, starting with a custom equal
function that checks the equality of two values while providing clearer logging for test failures. The lesson also introduces the use of Go's reflect
package to handle complex types, such as slices, that cannot be directly compared. This combination of a dedicated assertion function and reflection not only simplifies the assertion process but also improves the readability of error messages during testing. Furthermore, the necessity of handling error checks within tests is highlighted, advocating for the creation of a specific assertion function to manage error existence checks efficiently. Overall, these strategies contribute to more organized and effective testing practices in Go.
No links available for this lesson.
Throughout both this module and the course so far, we've written a decent number of tests.
However, one thing that's been tedious, at least in my opinion, has been when it comes to writing our test assertions. If we take a look at some of the assertions that we've written so far, you can see that they follow a very similar pattern, checking the equality of two values, and if they're not equal, logging out our expectation versus what we received, and failing the test calling the t.Fail
method.
Not only this, but whilst most of our assertions are pretty similar, they're not always consistent. For example, whilst most of our loglines are expected value and got value, in some cases we've accidentally diverged, such as once and got, as well as adding in a prefix string.
Because of how similar these test assertions are, and because of how tedious it is to write them over and over again, it's therefore a very common practice in Go to implement a test helper, in order to make writing these assertions easier. So, in this lesson, that's what we're going to go ahead and do, creating test assertions using two different approaches.
In order to begin, let's go ahead and create a new directory inside of our test directory, called assert
, which is going to be the package that we use for our test assertions. Then, inside of this directory, create a new file inside called equal.go
, which will host our first test assertion. Inside, let's give this the package name of assert
, and define a new function called Equal
, which is going to be the first assertion that we write in order to check the equality of two values. And we can pass in our two values as follows. The first is going to be our expectation, or our wants, which we'll define as any
, and the second value is going to be our got, or the result that we actually received. Again, go ahead and set this to be the any
type. For reference, the any
type is an interface that defines pretty much any type.
package assert
import "testing"
// Equal asserts that the want value is equal to the got value.
func Equal(t *testing.T, want any, got any) {
if want == got {
return
}
t.Helper()
t.Logf("want: %v\ngot: %v", want, got)
t.Fail()
}
To begin, let's go ahead and define a new function called Equal
. With our function signature defined, the next thing we want to do is go ahead and check to see if the two values are equal, which we can do as follows. If want
is equal to got
, then in this case, we just want to return early. If they're not equal, then we're going to want to fail our test. However, in order to do so, we're going to need to have access to our testing.T
type, which is what we currently use in order to both fail the test using the t.Fail
method, and to also print out our expectation string. Therefore, let's go ahead and modify the function signature of our Equal
function to first accept a parameter of testing.T
as follows, and we'll make sure to import the testing package.
With the testing.T
type now available to our function, let's go ahead and implement our Logf
, passing in the expected, and we use the v
verb so that we can specify any value, followed by got
, and again, we use the v
verb. Then we can pass in our wants and our got string, followed by then failing the test using the t.Fail
method. With that, our test helper should now be ready to go.
To test it out, let's head on over to our count test file, and make a change to one of our unit tests. Here, I'm going to go ahead and replace our test assertion in the TestAddCounts
function with this new method. So let's scroll down and replace this code, which I'm actually going to comment out for the meantime, with a call to assert.Equal
, passing in our testing.T
, then we can pass in our tc.want
and our actual results. Now, if I go ahead and make a change to the actual test expectation, just so that this test fails, we'll change this to be the value of three.
Now, when we go ahead and test this code, we should see the test fail, which it does. However, there's a couple of things I want to quickly change. The first thing is our expectation is currently on a single line. It's going to be a little easier for us to read if we split each of our expectation and our actual result onto their own lines. So let's go ahead and do so. Heading on over to our assert.equal.go
file and adding in the following new line character before the expectation and a new line character before the got
.
package assert
import "testing"
// Equal asserts that the want value is equal to the got value.
func Equal(t *testing.T, want any, got any) {
if want == got {
return
}
t.Helper()
t.Logf("want:\n%v\ngot:\n%v", want, got)
t.Fail()
}
Now, if we go ahead and test this code again, you can see it is a little easier to read. However, I think it would be even easier if we actually aligned the value columns so that we can just look down and see what's actually differed between the two. In order to achieve this, we just need to add in five spaces before our got
string. Now, when I go ahead and test this code again, you can see that our values now line up and it's a little bit easier to see what has actually changed. In this case, the value of three.
package assert
import "testing"
// Equal asserts that the want value is equal to the got value.
func Equal(t *testing.T, want any, got any) {
if want == got {
return
}
t.Helper()
t.Logf("want:\n%v\ngot: \n%v", want, got)
t.Fail()
}
Whilst it's a little easier to see, we actually have another issue, however. In order to see what the problem is, if I revert the call to using the assert.Equal
function and go ahead and remove this package and then run the code again. If we ignore the changes we made to the expected and got string and instead focus on the actual file, you can see what the issue is. In our test assertion, the failure is being reported on the equal.go
file at line 10, which is where the code is being called inside of the equals
function. However, this doesn't give us an indication of where the test is actually failing, which our previous implementation actually did. For example, we can see here that the test is failing on the count test.go
file at line 363, which if we head on over to that file and jump to 363, we can see where the test, we can see gives us an indication of where the test is actually failing.
Whilst thanks to table-driven tests, we do have the actual test name and sub-test name that we can look up, it would still be nicer to know where exactly in the actual file the test failure was occurring. Fortunately, we can still provide this information when using a test helper, as the T
type of the testing package provides the Helper
method, which, if we take a quick look at in the documentation, says that the Helper
method marks the calling function as a test helper function, which means when printing file and line information, that function will be skipped. Therefore, let's go ahead and make use of this inside of our actual Equals
assertion. To do so, all we need to do is call the T.Helper()
function at the top of the function as follows.
package assert
import "testing"
// Equal asserts that the want value is equal to the got value.
func Equal(t *testing.T, want any, got any) {
t.Helper()
if want == got {
return
}
t.Logf("want:\n%v\ngot: \n%v", want, got)
t.Fail()
}
Now, if we go back to the count test.go
function and go back to using the assertion. Now, if I go ahead and test this code again, this time you can see we're getting the actual file where the test failed as well as the line number, rather than it pointing to equal.go
line number 10. So far, our test helper is working quite nicely and we can go ahead and replace all of the existing assertions with it.
Before we do so, however, we have yet another issue. Although it won't affect us in our current test cases, but it may affect us in our future ones. To show what this issue is, if I create a new test function called TestSomeStuff
and pass in the testing.T
as follows, then if we call our assert.Equal
function passing in the testing type and if we pass in a slice of int
of one, two, three and we'll test it and we'll compare it against another slice of int
called one, two, and three. If we go ahead and run this code as follows, you can see we actually get a panic. This is because we're trying to compare two uncomparable types. In Go, you can't just use the double equals in order to compare two slices to each other. This is a bit of an issue as we want our assertion function to be able to handle pretty much any type we throw at it.
In order to do so, we have two different approaches we can take. The first is to handle this at compile time, which would be to make use of generics in order to only pass in comparable values. This would look as follows. First, setting a generic type within our actual Equal
function, which we can define as T
and then making sure that this type was constrained to the comparable
interface as follows. Then we can replace our calls to any
with a call to T
instead. Now, if we try to run our code, you can see we no longer get a panic, but instead we get a compilation error, letting us know that the slice of integer does not satisfy comparable.
package assert
import "testing"
// Equal asserts that the want value is equal to the got value.
func Equal[T comparable](t *testing.T, want T, got T) {
t.Helper()
if want == got {
return
}
t.Logf("want:\n%v\ngot: \n%v", want, got)
t.Fail()
}
Whilst this helps to prevent our test code from panicking, it's still rather limiting in its approach. Instead, it would be nicer if we could find another way so that we can actually test slices of integers. Fortunately, the Go standard library actually provides a package for us to be able to do this, which is the reflect
package. The reflect
package is somewhat controversial when it comes to Go, as it implements something called runtime reflection, which allows a program to manipulate objects with arbitrary types. Reflection is a form of metaprogramming when it comes to programming languages, and allows an application or code to modify its own types or constructs. When it comes to Go, reflection should be used pretty sparingly, and there's a really good blog post called The Laws of Reflection written by Rob Pike back in 2011, which talks about how reflection can be very confusing and how it should be used when it comes to Go.
In most cases, I would recommend not using reflection. However, when it comes to testing, there's a bit of an exception to be made. This is because the reflect
package provides the DeepEqual
function, which allows us to easily compare whether or not two values are deeply equal. This definition is that two values are of identical type, so a slice to a slice, so a slice of int to a slice of int, or a slice of string, are deeply equal if one of the following cases applies. Values of distinct types are never deeply equal, meaning that an integer would never be deeply equal to a string, or vice versa, etc, etc. In this case, you can see that it provides some different rules for different types, such as array values, struct values, function values, interface values, map values, pointer values, and slice values. Slice values are deeply equal when all of the following are true. They are both nil or both non-nil, they have the same length, and either point to the same initial entry of the underlying array, or their corresponding elements up to length are deeply equal.
Therefore, what this means is we can use this DeepEqual
function in order to support types that aren't comparable by default. So rather than using a generic, which is a really nice approach, but doesn't actually provide as much functionality, let's instead make use of reflection. To do so, we first need to import the reflect
package, which we can do as follows. Then rather than using the double equal sign in order to check for equality, let's replace this with a call to if reflect.DeepEqual
, and we'll do the want
and got
. Then if these are equal, we'll return early.
package assert
import (
"reflect"
"strings"
"testing"
)
// Equal asserts that the want value is deeply equal to the got value.
func Equal(t *testing.T, want any, got any) {
t.Helper()
if reflect.DeepEqual(want, got) {
return
}
t.Logf("want:\n%v\ngot: \n%v", want, got)
t.Fail()
}
Now, if I go ahead and call the go test
command again, this time our tests should both run and we shouldn't receive a panic, which is the case. If we head back on over to our actual count test and change the value of this slice to be something different, we can see now that our assertion is working for testing the int slices, as well as printing out the respective values of both the expectation and what we received.
With that, by using the reflect
package, we've managed to add in more functionality to our test assertions. And we're now in a good place to go ahead and actually refactor our code to make use of it. So to do so, I'm going to go ahead and copy the assert.Equal
line and we'll go ahead and make the following changes to use it. First, let's go ahead and replace all of the functions inside of our unit tests or inside of our table-driven tests, which are our unit tests, passing in res
as follows. Again, we want to use res
. We'll just copy that and scroll up as well.
We have quite a few tests. It would have probably been a little nicer to do this a bit sooner, just so that we wouldn't have had to write all these expectations out. Okay, let's go ahead and test that the counter test is working fine, which it almost is. We've got this failure here. That's because we changed it at the start. Now, if we test it again, our count test should be fully migrated over, which it is. Now we can go about migrating our end-to-end tests and we'll begin with the multi-test file, which again is going to be pretty easy. We need to import the assert package and there's no test cases here, so it's just a once. I think that's all for this one.
Okay, now we've done the multi-test. Let's head on over to our single file test. However, here you'll notice we actually have a slight difference when it comes to our logging output. In this case, we're prefixing our log message with the string standard out not expected
. Whilst we could just ignore this for the current test case, it would be nice to have the ability to add this prefix string. And in the case of our no file test, here it's actually going to be required given the fact that we have two test assertions that we're making and we want to prefix what each of these tests is actually doing.
In the case of this test, it's testing for standard error. and in the case of the test on line 40, it's testing for standard out. Therefore, let's go ahead and make a change to our actual test function so that we can pass in a message string, which we could do as follows. However, if we do this, we now have an issue where the tests that we've already changed over suddenly no longer work as we now need to provide a string to them. However, we don't want to go ahead and make changes to these individual tests in order to produce a new string. This is because these tests actually don't want to provide a message and so we would end up needing to provide say an empty string but we really just want to omit it. If this sounds familiar, that's because we've actually seen this problem before in an earlier module when we were passing in the file name to our count words function.
The way we solved this was by using a variadic parameter which in this case makes a sense for us to do yet again. So if we head on over to our assert function, let's go ahead and make the actual messages a variadic parameter as well. Now in order to print out these messages, we can go ahead and just iterate over them, say m equals range of messages
or message
then we can just do a t.Logf
print out a new line character and use the %s
verb passing in the actual message as follows.
package assert
import (
"reflect"
"strings"
"testing"
)
// Equal asserts that the want value is deeply equal to the got value.
func Equal(t *testing.T, want any, got any, messages ...string) {
t.Helper()
if reflect.DeepEqual(want, got) {
return
}
message := strings.Join(messages, " ")
if message != "" {
t.Log(message)
}
t.Logf("want:\n%v\ngot: \n%v", want, got)
t.Fail()
}
Now if you head back on over to our no file test where we're making use of this function, let's go ahead and replace our two assertions with this assert.Equal
function, passing in our t
type then we want once std error
and std error.string
as well as passing in the actual message of std error doesn't match
, let's say. Then we can go ahead and do the same thing for our standard output stream t once standard out
standard out string
followed by standard out doesn't match
perfect.
If we go ahead and just make sure that these tests fail, we'll just put in a fail or break, let's say, and then bad test. Now if we go ahead and run these tests using the following test/e2e
, as you can see, we now get some additional outputs with our error message. However, this to me is kind of difficult to read as you can see, we're getting the file name and line being printed multiple times which doesn't really make sense and makes it seem like there's multiple tests failing when in reality there's only one. This is happening because we're calling the t.Logf
function multiple times and if we happen to pass in multiple messages, say multi
as follows, then this looks even worse as you can see we're seeing no file test.go 36
appear three times.
Therefore rather than calling the Logf
method of the testing.T multiple times, we instead just want to call it once passing in all of our messages as a string. Fortunately, we can achieve this by creating a message string using the join function of the strings package and in this case we'll just go ahead and join it with say an empty space as follows. Then when it comes to printing this out, we can use the following verb of %s
and we'll just pass in the message string. Now if we go ahead and run this again, we should no longer see the no file test.go 36
appear multiple times and same for the 37
string as well, and we should only see it appear once with all of our messages on the same line.
As you can see, we get standard error doesn't match multi
and then we get our two expectation strings and having the new line makes it a little easier to see in between each test. With that, we've managed to add in support for messages in order to provide additional context as to what has actually failed. So let's go back to our no file test and we can remove the conditions causing our test to fail and let's also remove the additional message of multi
here as well and then we can go ahead to our standard input test where we have the same thing standard out is invalid not correct
and let's go ahead and import the assert package.
And I believe that is everything now all of the tests should be making use of the new assert package which if I go ahead and run the following command to test them all and we use the -v
, they should all be passing which they are. With that, we've managed to reduce some of the tediousness of our code by implementing a new test assertion function in order to assert the equality between two values that we pass in. Additionally, we also made use of the reflect package in order to support quality checking between types that don't normally conform to the comparable interface in Go. However, this isn't the only assertion that we're making when it comes to our test cases with the other assertion that we're often making being to check whether or not an error exists with the other common assertion that we're often making being to check for the existence of an error.
And in the case that it does exist, we're calling the t.Fatal
function causing our test to fail. Therefore before moving on to the next module now would be a good idea to spend some time creating a specific test assertion in order to check in order to handle this if error check and if the error is not nil calling the t.Fatal
function where you add this new assertion function in.
Once you've done so, make sure to go ahead and commit your code to git and I'll see you in the next module.