Please purchase the course to watch this video.
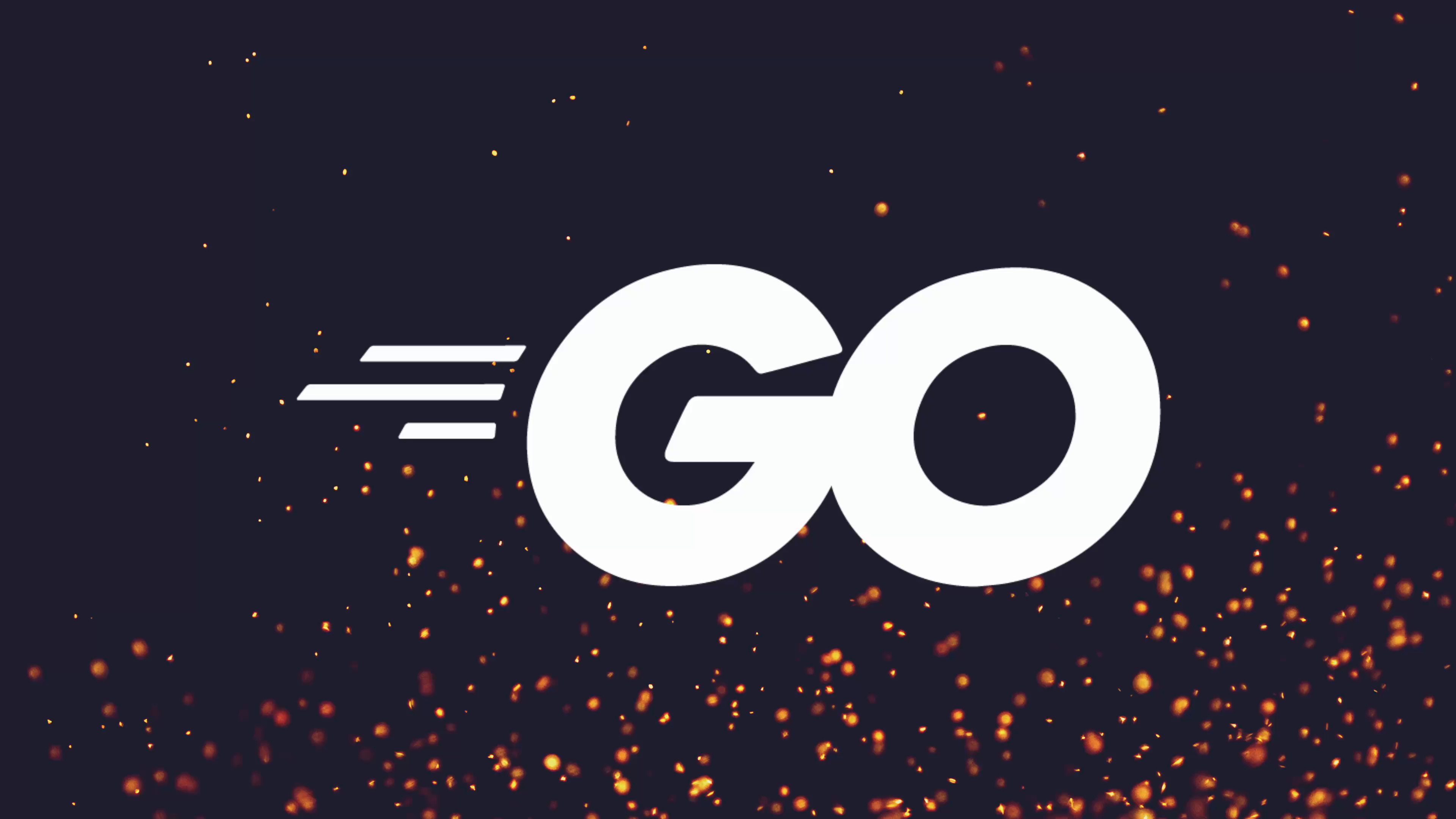
Full Course
The counter application has evolved to effectively process input files, displaying results for words, lines, and bytes accurately. However, a subtle output formatting issue arises, particularly when handling varying file types, including empty files. In contrast to the wc
command, which aligns its output neatly in a tabular format, the current implementation shows staggered results. To enhance readability, the Go Tab Writer Package
is introduced, which allows for improved column alignment using the ElasticTabStop
algorithm. By modifying the code to incorporate this package, including adjusting parameters for minimum width and tab widths, the output can achieve right-aligned numerical columns while maintaining left-alignment for filenames. Furthermore, lessons emphasize the importance of flushing the writer to avoid data loss during execution and provide an assignment that challenges learners to implement a header for better context in output results. As the module concludes, learners are prepared to explore more advanced features of Go, including concurrency and end-to-end testing.
No links available for this lesson.
Currently, our counter application is in a good spot. If we run our code, passing in a file like words.txt
, you can see it works correctly. It's also working for standard input — for example, when we pipe the output of another command into it.
However, we do have a minor issue. If we run:
go run . words.txt lines.txt
You’ll notice a subtle problem: our output is slightly staggered. Compare this with the standard wc
command:
wc words.txt lines.txt
You’ll see that wc
aligns the numbers in neat columns, even with varying digit lengths. For example, when we add an empty file using:
touch empty.txt
go run . words.txt lines.txt empty.txt
the staggering worsens. Adding a large file like lots-of-words.txt
also causes visual inconsistency. In contrast:
wc words.txt lines.txt empty.txt lots-of-words.txt
outputs everything in a tabular format, with right-aligned columns — making relative comparisons easier.
Introducing the text/tabwriter
Package
Go provides a standard library package to help with this: text/tabwriter
. It implements a write filter that translates tabbed columns into aligned text using the Elastic Tab Stop algorithm.
Adding the Tab Writer
Start by importing the package:
import "text/tabwriter"
Then, inside main()
, create the writer:
wr := tabwriter.NewWriter(os.Stdout, 0, 8, 0, ' ', 0)
Here’s a breakdown of the parameters:
os.Stdout
: Output destination.minWidth
: Minimum cell width. We’ll use0
.tabWidth
: Width of tab character. We'll set this to8
.padding
: Extra padding before computing width. Set to0
for now.padChar
: The character used for padding. We'll use a space' '
.flags
: We'll use0
for now, but can update later.
Now replace your output destination (e.g., os.Stdout
) with wr
.
Fixing Alignment with Tabs
We're not seeing results yet because we’re still joining with spaces. Replace that with tabs (\t
). For example:
line := strings.Join(stats, "\t") + "\n"
Still not working? That’s because tabwriter buffers internally, so we must call Flush()
:
wr.Flush()
Don't defer Flush()
if you call os.Exit()
, as defer
won’t run. Instead, explicitly call it before exiting.
Adding Padding and Aligning Right
To make the alignment better:
wr := tabwriter.NewWriter(os.Stdout, 0, 8, 1, ' ', tabwriter.AlignRight)
Now the numbers will be right-aligned. But the filenames are also right-aligned — which we don’t want. We want filenames left-aligned like wc
.
Fixing Filename Alignment
To fix this, avoid appending the filename in the same tabbed line. Instead, output it after the tabbed numbers:
stats := []string{lineCount, wordCount, byteCount}
line := strings.Join(stats, "\t") + "\t"
if filename != "" {
line += filename
}
fmt.Fprintln(wr, line)
This way, your stats will align right, and filenames will stay left-aligned.
Updating Tests
Because we’ve added tabs, we need to update the expected output in count_test.go
. For example:
expected := "5\t0\t24\t\n"
Add tabs between numbers, and make sure tests pass.
Handling Edge Cases
There’s a subtle issue when filename
is empty — we might accidentally include a trailing space. Fix it like this:
fmt.Fprintf(wr, "%s", line)
if filename != "" {
fmt.Fprint(wr, " " + filename)
}
fmt.Fprintln(wr)
Assignment: Add a -header
Flag
Add a CLI flag -header
that prints column headers like:
Lines Words Bytes
12 5 100
Only include the headers for the enabled columns (-c
, -w
, -l
). You may omit the filename header if standard input is being used — or optionally include it. The header should only show when -header
is passed.
Test behavior:
go run . -header words.txt
Outputs:
Lines Words Bytes
12 5 100
Or with constrained flags:
go run . -header -w words.txt
Outputs:
Words
5
This wraps up this module! In the next module, we’ll explore advanced Go features like concurrency and end-to-end testing.