Please purchase the course to watch this video.
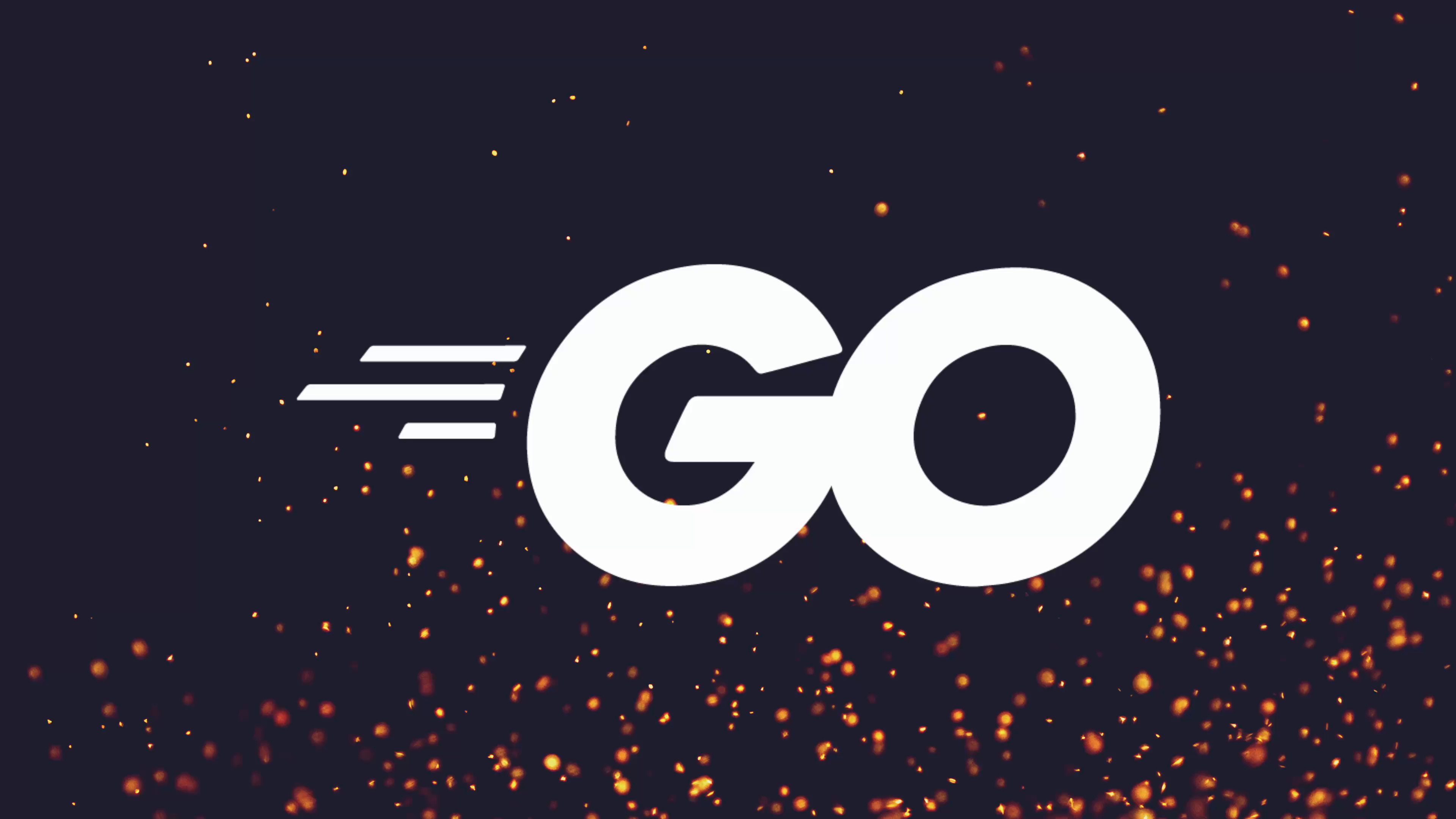
Full Course
The ability to interact with network resources is crucial for building robust CLI applications, and the Go standard library offers effective tools for this purpose through its net
package. This package facilitates the creation of TCP servers, enabling developers to establish and manage connections seamlessly. By utilizing functions like net.Listen
and net.Dial
, one can create a simple echo server that accepts client connections and responds by echoing back any received data. The lesson highlights essential practices like error handling, utilizing goroutines for concurrent connection management, and leveraging the io
and bufio
packages for efficient data transfer and logging. It also touches on more advanced functionalities available for fine-tuning connections, such as ListenTCP
for tailored server settings, underscoring the flexibility and power of Go's networking capabilities.
No links available for this lesson.
Throughout this course, we've been building both features and functionality related to working with data on the file system, or provided through user input. In addition to these, another common source of data interaction for CLI applications is the network, whether it's your own local network or via the internet. When it comes to networking, the Go standard library provides a number of different packages that allows us to interact with the networking stack on our local machines. One of these is the net
package, which provides basic primitives for working with networking in Go.
If we take a look at the documentation for this package, we can see it gives some examples on how we can connect to a TCP server using the net.Dial
function, or how we can create our own TCP server using the Listen
function of the net
package. Let's take a look at how we can use this package to create our own simple TCP echo server. To do so, I'm going to create a new directory called echo
and initialize it using the go mod init
command.
Then let's open up the main.go
package and define our main function. Then in order to create a TCP server, we can use the net.Listen
function as we saw in the documentation examples. You'll notice that there are actually a number of different Listen
functions we can use, including specific ones for TCP and UDP. We'll take a look at the TCP one later on. However, for the meantime, let's just use the basic Listen
function. This function returns two different types: a listener, which we can define as lr
, and an error, in case it can't bind to the address for whatever reason.
Let's do a quick error handling of log.Fatal
line failed to listen on TCP 8080. And we'll do an fmt.Println
server listening on port 8080. In fact, no, this is going to be a little bit too big, so let's reduce it down. With that, our TCP server should now be running on port 8080. The next thing we want to do is add in code to accept connections when a client attempts to connect.
If we take a look at the documentation example, you can see that this is done inside of an infinite loop. So let's go ahead and do the exact same thing. First, defining an infinite for loop as follows, then collecting the connection and an error using the lr.Accept
method. Let's also handle the error in case something goes wrong. In this case, we can just do a continue
function with an fmt.Println
failed to connect. Then let's go ahead and use the defer
keyword to close the connection at the end of the file. And we'll do an fmt.Println
letting us know that a client was connected. And let's print out the connection's remote address string.
Okay, with our TCP server code added, let's go ahead and run this code using the go run
command. And we can see that the server is listening on port 8080. Then in order to test our client connection out, we can use the telnet
package, which I don't actually have installed on my machine. Therefore, let me go ahead and set up a Nix shell to use one:
nix-shell nixpkgs.telnet
Which doesn't exist, so I need to use busybox
. Then I can go ahead and telnet
to the address we've defined on port 8080. As you can see, I'm now connected to my server and I received an event letting us know that we've connected on port 60896, which is the remote address that this telnet service is using.
So let's go ahead and exit this and add in some code so that we can actually echo back whatever data we receive from the client back to it. To do so, we're going to need to use a go routine in order to make sure that we can handle multiple connections at the same time. So we can start one up using the go func
command as follows. Next, we then want to be able to pull out the data from our connection and send it back to it. If we take a look at the actual net.Conn
type inside of our documentation, you can see that it actually conforms to both the io.Reader
and io.Writer
interfaces. This means it's actually incredibly easy for us to create an echo server. We can just use the io.Copy
command to copy the connection reader to the connection writer.
Now if we go ahead and run this code and create another telnet by first using:
nix-shell nixpkgs.busybox
Okay, now I can connect. Now if I send a message to the server, "hello", I get a message back. With that, we have a really simple echo server up and running. However, it would be nice to be able to see the messages come through on the actual server logs and to also prefix the word "echo" to them. Again, we can do this pretty easily by using the functionality of the io
package, of which we've seen already throughout this course.
Let's begin by first creating a multi-writer, so we can write to both stdout
and our connection at the same time. We can do this by using io.MultiWriter
, passing in the con
and os.Stdout
. Now we can go ahead and use the io.Copy
, passing the writer and reading from our connection. Now if I go ahead and run this code again, go run .
and telnet
back in, you can see now the output is appearing both in stdout
on the server and being written back to the client.
Pretty great. However, what if we want to add a prefix to each of these outputs of say "echo" and my actual message? So I can easily differentiate what I sent to the client and what I received back. In that case, we can use the bufio.Scanner
that we've already seen before. Let's go ahead and create a scanner as follows, passing in the connection as the reader. Then we can go ahead and iterate over the scanner using the for scanner.Scan()
method. Then we can write to our multi-writer using the fmt.Fprintf
method using "echo" and then scanner.Text()
.
Oh, let's pass in our writer as well. Now if we go ahead and restart the server and connect again using telnet
. This time, if I type in "hello", you can see we get "echo hello" and "echo hello" again. However, let's say on my TCP server, I don't want to have the prefix of "echo". Well, fortunately, we can solve this by getting rid of the multi-writer and just writing to both our connection and os.Stdout
as follows. Let's go ahead and get rid of the io
package as we're no longer using it. And then run our code again.
And voila! Everything is working as expected. As you can see, when it comes to passing data through both readers and writers, the io
package and the bufio
package have a lot of functionality that allow you to set up your data reading and data writing however you want it. Of course, however, this lesson is about the net
package. So let's take a look at some of the other features that it provides.
As I mentioned before, there's a number of other different Listen
functions that we can use, including ListenIP
, ListenTCP
, and ListenUDP
. In our case, we're listening to TCP. So let's go ahead and use the TCP
function to see what extra features we get inside of the net.TCPListener
type. To use it, we can no longer pass in a string. Instead, we need to pass in a net/TCP
address type, which is a struct. This struct takes two different properties, which is an IP and a port.
In our case, we can set the IP, which is a net.IP
as follows, using the net.IPv4
, passing in the individual bytes, in our case 127.0.0.1
. Then for the port, let's go ahead and pass this in as 8080
. As you can tell, this isn't as easy as passing in the address string that we did with the standard Listen
function. We get a net.TCPListener
type back, which has a number of other methods that we can actually use.
The first is an AcceptTCP
function, which specializes the net.Conn
type we receive, which typically comes back in the Accept
function. This is a net.TCPConn
, which, if we take a look at the documentation, has a few other methods associated with it, such as SetDeadline
, SetKeepAlive
, SetLinger
, etc. This is useful if you want to add custom configuration to the TCP connection that you're actually using. Additionally, the TCP receiver also has the SetDeadline
method, which allows you to set a deadline associated with the listener. This is useful for constraining the amount of time that you're going to listen to on the TCP server, which you can use in conjunction with cancellation in order to have graceful cleanup.
Therefore, if you're deciding to spin up your own TCP server, whilst you can just use the Listen
function, if you want more fine-grained controls, then it's worthwhile using the ListenTCP
method. And the same applies if you want to use any of the other IP-based protocols, including ListenUDP
, ListenIP
, ListenUnix
, Use ListenPacket
, ListenUnixgram
, or even ListenMulticastUDP
. Whichever you need, you should have. For the meantime, however, let's just leave this with the ListenTCP
function.