Please purchase the course to watch this video.
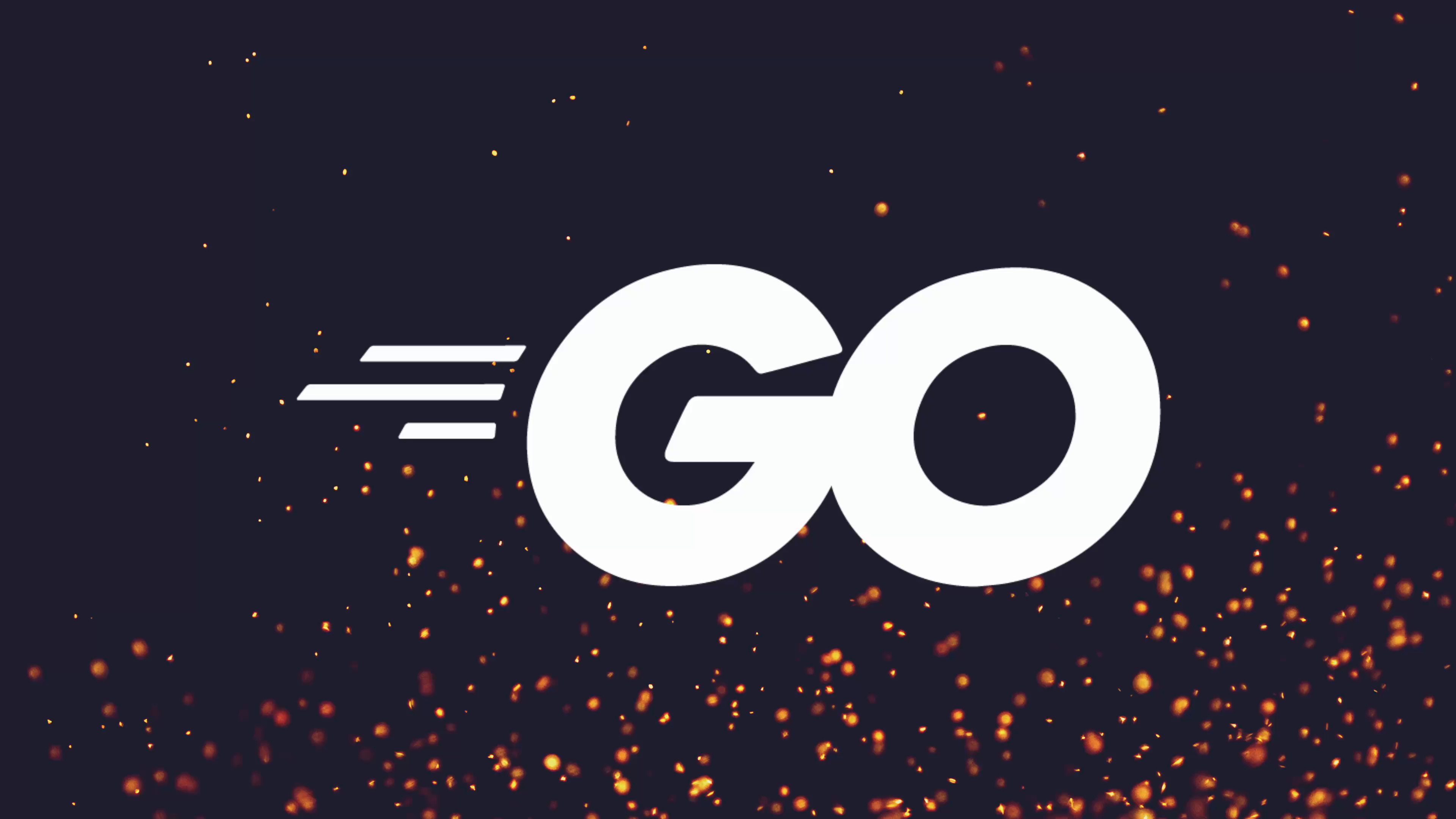
Full Course
The module delves into advanced file and network I/O operations in Go, emphasizing the significance of file flags and permissions for effective file management. It simplifies complex concepts like Unix file modes and explores advanced file operations such as lock files and PID files for process management. The lessons further navigate through networking protocols using the net
package, introducing the creation of TCP clients and servers, a DNS lookup tool, and a basic port scanner while highlighting important considerations. The focus then shifts to the net/http
package for building HTTP applications, covering data encoding, file uploads, and testing HTTP functionality using the HTTP test
package. This comprehensive exploration equips developers with essential tools and techniques for robust application development involving file systems and network communications.
No links available for this lesson.
In this module, we're going to be talking a lot about I/O, specifically, networking and the file system. We'll begin the module by looking at some advanced ways of working with files, first beginning with file flags, which allow us to modify the behaviour of creating or opening a file, as well as constraining whether we can write, read or do both with it.
Afterwards, we'll then jump into Unix file permissions, also known as file modes. This is something that can feel rather arcane when getting started building CLI applications, but it's actually a lot easier than one might initially think.
Once we've taken a look at how we can both control files using file flags and file modes or file permissions, we'll then take a look at some more advanced file operations when it comes to Go, things such as using lock files or PID files to prevent multiple applications running at the same time, and even making use of files as a sort of mutex, through the Flock
system call.
Additionally, we're also going to take a look at how we can actually walk the file system, which will allow us to create applications that can perform recursive actions. For example, we'll take a look at how we can find all of the files ending in a certain suffix or a certain extension inside of a large directory.
After we've taken a look at files, we're then going to move on to networking protocols, through the use of the net
package provided by the standard library, beginning with creating a simple TCP client and server to echo commands whenever we send data to it.
Afterwards, we'll then move on to some more advanced TCP concepts, such as creating a DNS lookup tool, which we can use to resolve DNS records to an IP address, and do the inverse, where we can perform a reverse DNS lookup, resolving an IP address to a DNS record.
After that, we're then going to move on to building a simple port scanner, which we can use to check for any open ports on our system or a remote system, as well as some of the caveats of doing so.
Once we have a good understanding of some of the things you can achieve using the net
package, we'll then move on to the net/http
package, which provides more specialism when it comes to working with the HTTP protocol, for things such as creating HTTP servers and creating HTTP clients, which we're going to create a few of, working with the HTTP bin service.
After we have a good introduction into HTTP, we'll then take a look at how we can actually encode data to files and to our actual network, looking at both JSON data, YAML data, as well as looking at form encoding, which is typically used when it comes to websites.
Afterwards, we're then going to blend both files and the network to look at how we can upload files using HTTP and multipart form encoding.
Then we're going to finish off the module by looking at how we can actually test HTTP request functionality in our code, using the fantastic HTTP test
package, provided by the standard library, which will allow us to easily spin up a test HTTP server that we can use to capture requests and send back dummy response, in order for us to be able to test our applications without needing to hit real services.
As you can tell, this is a pretty big module, and it covers a lot of advanced things. So without further ado, let's jump in.