Create an account to watch this video
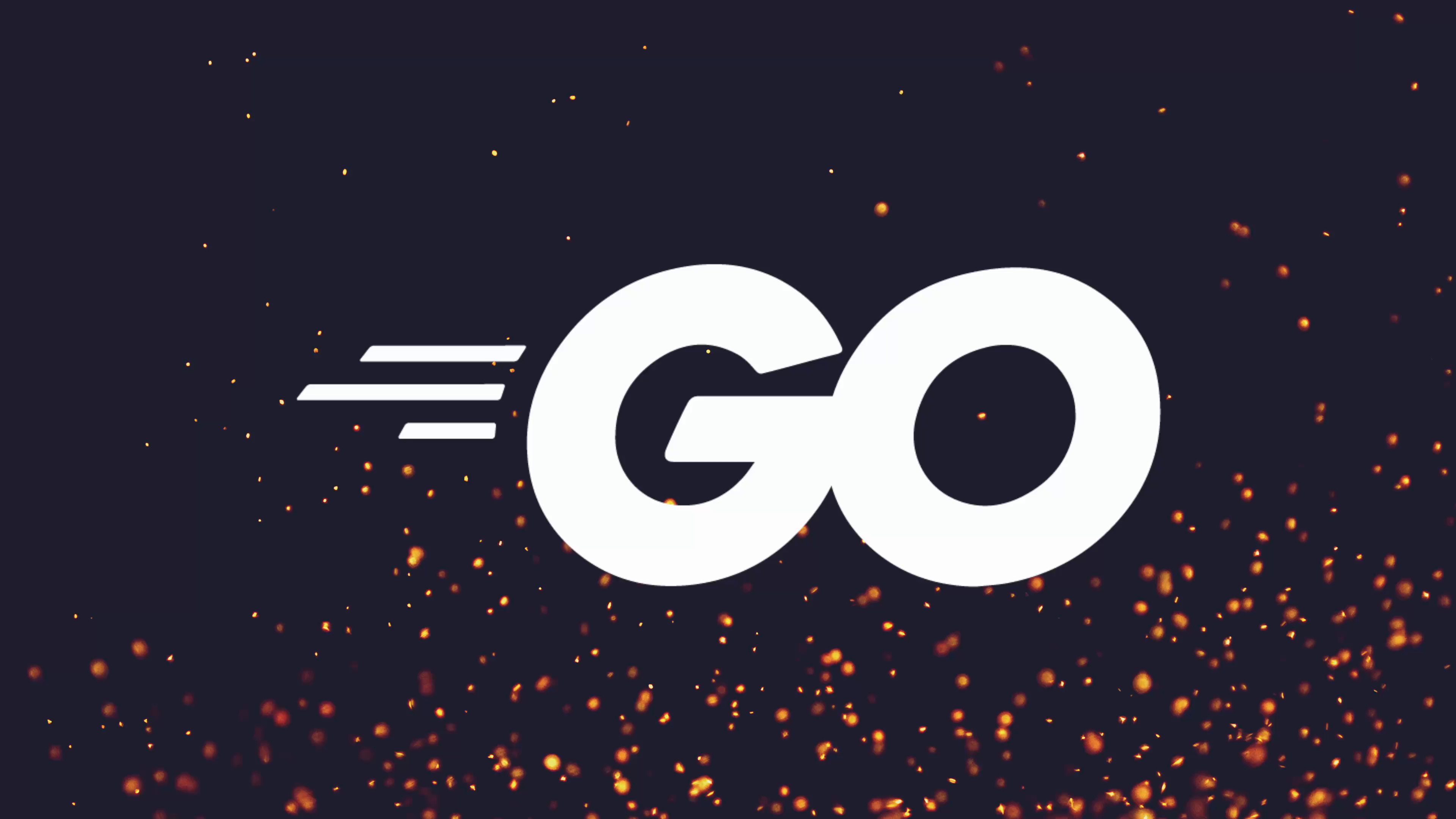
Full Course
Automated testing is crucial for ensuring code reliability during software development, particularly when modifying algorithms to handle edge cases, as highlighted by the counter application's current limitations. The session emphasizes the importance of unit testing, which allows developers to confirm that individual pieces of code, like the word counting algorithm, function as expected even after changes are made. By defining clear input-output expectations and creating a structured testing framework in Go, developers can efficiently catch regressions—issues where previously functional code fails after updates. The approach showcased includes setting up a count_words
function to isolate functionality for testing, thereby following best practices to enhance code robustness while preparing for the implementation of additional features.
So far our counter application is starting to come along.
By the end of the last lesson we'd managed to add in a simple word counting algorithm. That's if we go ahead and run against the following words.txt
, you can see produces the correct result of 5. Whilst this algorithm works for a well-formed sentence such as in the words.txt
file, it unfortunately doesn't work for a number of other cases.
For example, if I go ahead and modify the words.txt
to be an empty file, and if we go ahead and run the code again, you can see it produces the number 1, when instead it should produce the number 0. This empty file is part of what's known as an edge case, and is currently something our algorithm doesn't handle well. We'll take a look at edge cases more in an upcoming lesson in this module, as well as how we can modify our algorithm to be able to better handle them.
However, before we start making changes to our algorithm, we're first going to spend some time making sure that we don't introduce any new bugs when we start adding in new code. The process of adding in new bugs when you make code changes is known as regressions. This is where behaviour that was once previously working suddenly no longer works when you make a code change, and they can be quite a blight when it comes to software development, typically being one of the more frustrating experiences when you begin building out a larger project.
One of the best ways to prevent regressions from occurring in your code is to implement automated testing, which allows you to easily confirm that behaviour works even as you go about making code changes. For example, in our case, we could add in an automated test that will test our well-formed sentence to be sure that it will always print out the number 5. Therefore, before we start adding in some more complexity into our algorithm, now is a good time to go ahead and start implementing a simple automated test to ensure that our current behaviour works as is.
When it comes to software development, there are actually many different types of automated tests that you can implement, with the three major ones being integration tests, end-to-end tests and unit tests. We'll take a look at all of these throughout this course, as well as some performance tests later on as well, in order to determine which version of our algorithm performs the best. However, for this lesson, we're going to focus on unit tests specifically.
When it comes to larger software projects, unit tests tend to be the most common form of tests that you'll find. In fact, there's an old blog post from Google back around 10 years ago, which talks about the testing pyramid, which describes the amount of tests that your project would typically have. As they say, the bulk of your tests are unit tests at the bottom of the pyramid, and as you move up the pyramid your tests get larger, but at the same time the number of tests gets smaller. This is still reasonably good advice, at least in my opinion. Personally, I find unit tests to be essential for ensuring that your code works as intended, although there are some rules that I personally follow when it comes to writing unit tests to ensure that they are a benefit and not a burden. We'll talk a little bit more about what those rules are through this lesson and the next.
However, for the meantime, let's go about actually adding in some unit tests into our code. In order to do so, we first need to define what a unit test will actually test. Generally, a unit, when it comes to unit test, is defined as the smallest unit of code. However, that definition is up to interpretation, and is usually defined by the project maintainer or project owner. In our case, a unit is going to be a single function, and the single function we want to test in our case is going to be the actual word counting algorithm.
However, in order for us to be able to test this code, we need to make sure it's in a testable state, because at the moment, it's not. This is because all of our code lives inside of the main function, and unfortunately, the main function can only be called by the operating system, and we can't actually call it from within our actual code. Therefore, in order to make our code testable, we're going to need to create a new function in order for it to be able to be called by the test code.
So in order to make our code testable, let's go ahead and create a new function called CountWords
. This function will accept a slice of bytes []byte
as a parameter, which we'll go ahead and call data
as follows. This is the return value that we're getting from the os.ReadFile
function, and so it makes sense to set it to be a slice of bytes at this stage. Next up, we then need to go ahead and define a return value, which in this case is going to be a simple integer. This will be the number of words that we detect within the slice of bytes. With that, our function signature is now defined.
However, before we go about implementing the actual algorithm inside of this, let's just go ahead and return 0 for the moment. We will implement the algorithm, but before we do so, we're actually going to start implementing our tests. This is so we can be sure that our test code is working correctly, by it initially failing. Then once we go ahead and add in our algorithm, we can be sure that our changes caused the test to pass. This is very similar to a test-driven development approach, which is where you typically write the tests beforehand, before writing your implementation.
We're not going to be following TDD in this course, but there are some places where it will make sense, and this, in my opinion, is a good place to test it out in order to see some of the benefits. However, as I mentioned, we're not going to be doing TDD throughout this course. Instead, this is just a learning exercise to understand how we can test our code, and to make the changes to our algorithm easier to test in the upcoming lessons.
In any case, with our function now defined, we can go about actually writing our test code in order to call this function, and see if it returns the correct result. To do so, go ahead and create a new file inside of your project, called main_test.go
. In Go, any file that has the _test
prefix in its name is understood by the Go toolchain to be a test file, which means it will be executed when we run the go test
command, which we'll take a look at in a minute.
Then inside of this file, let's just go ahead and give it the package main
for the moment. When it comes to Go, every file inside of the same directory must have the same package name. Therefore, in this case, because we're inside of the main package, then this test file also needs to have the main name as well. There is one exception to this rule when it comes to test files. In those cases, you can give the suffix of _test
. We'll take a look at that more in the next lesson and the benefits of doing so. However, for this one, let's just go ahead and keep it as the same package, package main
.
Next up, we then want to go ahead and create a test function. In order to do so in Go, there's a required convention, which is to define the function name with the test_
prefix. This again tells the Go toolchain that this is a test function, which should be executed when again we run the go test
command. Therefore, let's go ahead and set the name of this function to be TestCountWords
, as we're going to be testing the CountWords
function.
Then for the parameter of this function, again there's a convention here that's required, which is to pass in a type of t
, which is going to be a type of testing.T
, which comes from the testing package, which I'll go ahead and import, and it's going to be of testing.T
. This defines a test. This testing.T
is a test type in the testing package. Next up, we can actually go ahead and write our test. When it comes to unit tests, for me, I like to think of them as being input and output. So you pass input to a function, and you receive output back. This is a very functional way of testing, and it helps to prevent any of the complexities that come about needing to set up your system or your environment into the correct state.
In our case, because our CountWords
function is very simple, all it does is take an input, which is a slice of bytes, and returns back an integer, then we can consider this function to be pure, meaning that any outside state won't have an effect on it. These are, in my opinion, the best sort of functions to test, as you can be guaranteed that anything you pass in will always be the same when it comes out.
In any case, in order to test this, we first need to define two properties. The first is our input, which we can define as input
. We're going to define this as "one two three four five"
as follows. With our input defined, the next thing we need to define is our expectation. This is what we expect to get back from the function call, given the provided input. In this case, I'm going to go ahead and define this as wants
, as this is what we want to get back from the function.
wants
is a bit of a Go term for the expectation, but I personally like it because it's five characters long, allows the input and once names to line up nicely. In any case, our expectation here is going to be an integer, which is what the return value of the count_words
function is. So let's go ahead and set this to be once = 5
. This is because there's five words inside, so we're expecting five words back.
Okay, with both our input and expectation defined, the next thing we need to do is obtain the result. In this case, we can go ahead and say res
, but you could also call it something like gots
, or anything else you like. Personally, I like to call it res
as shorthand for the result. You could also call it result
as well if you want to. Let's call it result
, just to be a bit more explicit. res
is what I tend to prefer, but result
also works as well. In any case, with our result variable defined, we can then go ahead and assign it to the return value of the CountWords
function, passing in our input
.
Unfortunately, however, when I do so, you can see I actually get a compiler error, or at least my editor is letting me know that an error will occur. This error is cannot use input variable of type string as a slice of byte values into the argument
. This is because our CountWords
function accepts a slice of byte, and not a string. Fortunately, this isn't too difficult to solve. This is because, similar to how we were able to cast a slice of bytes into a string, we can use the same syntax in order to cast a string into a slice of bytes, which is by first defining the slice of byte type and wrapping our input
with it.
With that, we can now call the CountWords
function with our input string. However, this time we are getting back some different errors, due to unused variables. So, let's go ahead and actually use them. To do so, the next thing we're going to need to implement is an assertion. Every test should have at least one assertion, which is where you're checking the result meets your expected. And if it doesn't, then you want to fail the test.
In Go this is done using a simple if statement. So, if result
is not equal to want
, which is our assertion, that means it's failed. In order to fail a test in Go, we can use the t.Fail
method of the testing.T
type, which if we read the documentation, marks the function as having failed, but continues execution. There are a number of other ways to fail when it comes to this testing type, such as t.Fail
and t.FailNow
. The difference between t.Fail
and t.FailNow
is actually within the name. t.FailNow
marks the function as having failed, and stops its execution. This differs from the Fail
function, which will still continue the test even after it's been called.
There are some legitimate reasons to use t.FailNow
, but most of the time I think using a t.Fail
is more appropriate, as you may still want other assertions to also take place, so that you can see what else may be failing. This makes it a little easier to go ahead and fix your code, rather than have a run your test, see it fail, fix that one failure, then see it fail again. Personally, I think it's much nicer to see all of the failures at once, then I can go ahead and fix each one.
In any case, let's go ahead and call the t.Fail
function. And with that, our test is now ready to go. Let's go ahead and actually test our code to see how it looks. In order to test code using Go, you can use the go test
command, passing in the directory that you want to test. In this case, we can just use the dot syntax, which when I do, you can see runs the test, the test count_words
, which is now failing, as we're expecting.
This is failing because we haven't implemented the actual code. In addition to using the go test
command with the dot, which means the current directory, if you have a larger project, you can actually run all of the tests by using the following syntax. .
for the current directory, /
, and then ...
, which means all of the subdirectories within that directory. Again, we only have a single directory at the moment, we'll take a look at structuring our code later on throughout this course, but for the meantime, this works for our current setup.
As well as using the go test
command, you can also use the -v
flag, which stands for verbose. This will provide more output for your actual test cases, specifically showing you each individual test case, whether it's passing or failing, as well as any log output you may be printing inside. We'll take a look at that more later on throughout the course. Currently, it doesn't make any difference to our output, given the fact that all of our tests are failing, so we're getting the verbose logging already.
In any case, as we can see, our go tests
are failing, which means we can now be certain that any code changes we make to fix this code will work. So to do so, let's head back on over to our main.go
function, and go ahead and implement our algorithm in order to be sure that our test is working. To do so, let's just go ahead and add in the same code that we had before, which was defining a word count, which was set to be zero, and returning it at the end.
Then, for the actual algorithm itself, we can go ahead and do a for range over our data
slice, as we were doing before, checking to see if the byte value is a space, and then just incrementing our word count ++
. Lastly, we then need to increment our word count by one, in order to handle the delta between the number of spaces and the number of words, so let's go ahead and do so.
Now, if we go ahead and test our code again using the go test
command, you can see that it's now passing, meaning that we can be certain that our changes caused this test to pass. Before we move on, the last thing we need to do is to go ahead and actually replace the code inside of our main function in order to make use of this new CountWords
function.
So in order to do so, let's just go ahead and delete the following code, and we'll go ahead and we'll also go ahead and delete this line where we were making sure to squash any errors regarding our data
variable. And we can go ahead and replace all of this with wordCount
, assigning it to the return value of CountWords
and passing in our data
.
Okay, with that, our code should also be working when we run the go run
command, and now it's making sure to also call the count_words
function. Although one thing to be aware of, this is one of the limitations of unit tests. Whilst we can be sure that our CountWords
function is working correctly, we can't ever be too sure that our main function is working correctly.
In order to do that, we need to use end-to-end tests, which we actually have a dedicated module on advanced testing later on, which does go into how to end-to-end test a command line application from start to finish. In any case, now that we have our first test implemented, we can be sure that any changes we make to this code won't cause this test case to fail.
However, before we start making any more changes, in the next lesson I want to talk about how we can actually make these tests less brittle, whilst also then beginning implementing our first edge case. So before we do that, let's go ahead and check our git status
, which shows us that we have changes to our main.go
and also a new main_test.go
file to add to our git repository.
So let's go ahead and do so using the git add
command, adding in the main.go
file and adding in the main_test.go
file. Then we can go ahead and write the following commit message using git commit -m
, the -m
stands for message, just in case I didn't mention that earlier. Here we can go ahead and write added in first test case and created new count_words function
.
And with that, our code is now committed and we're ready to move on to the next lesson.