Please purchase the course to watch this video.
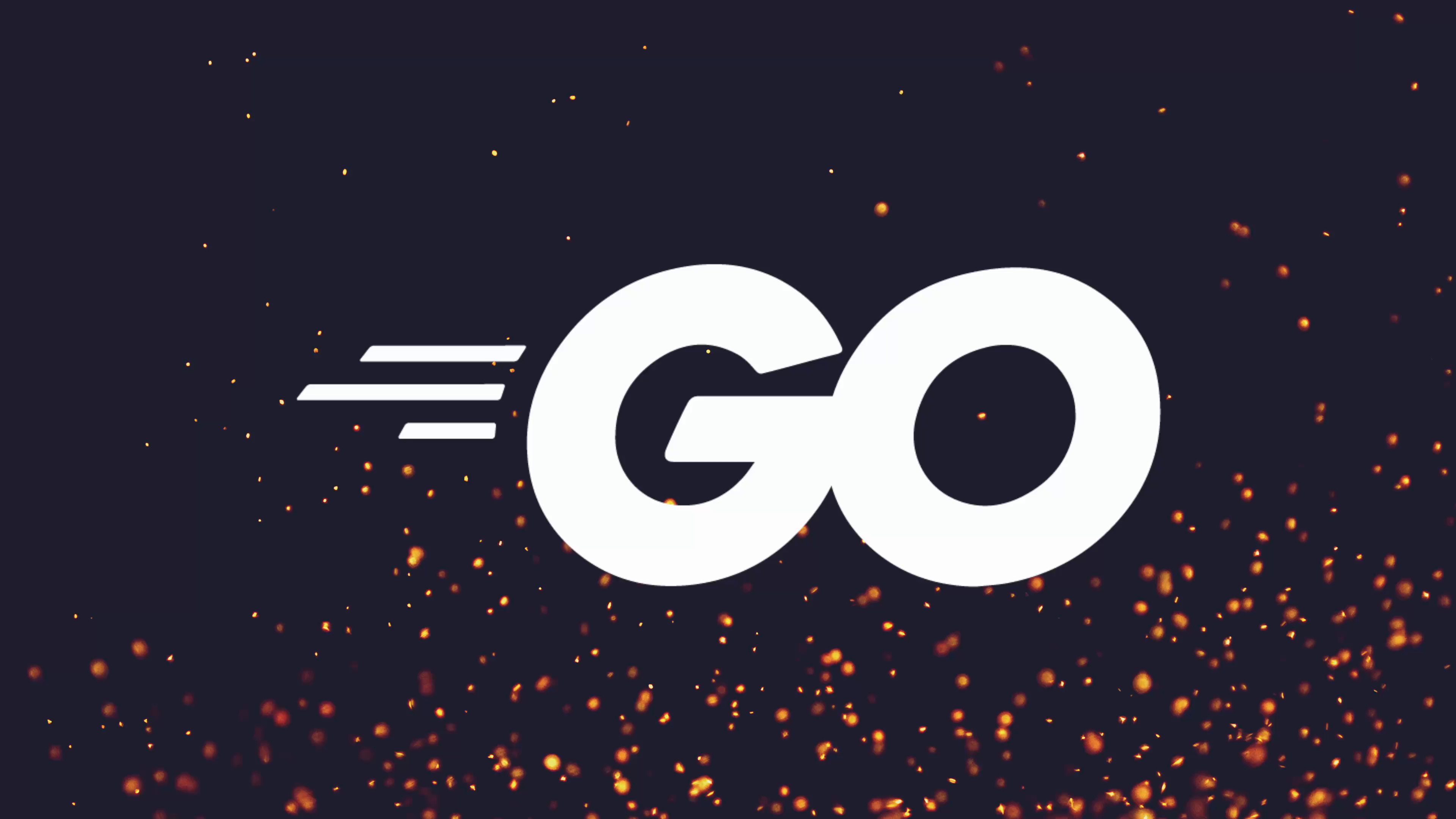
Full Course
Uploading files is a pivotal operation in HTTP communication, enabling tasks like profile picture uploads or file storage on remote servers. When creating command-line applications, the capability to handle file uploads is equally essential. This process typically utilizes POST requests, encoding data in various formats, such as JSON or HTTP forms. However, to effectively manage file uploads, a multi-part form data request is necessary, which allows different data types—including text, images, and binary files—to coexist within a single HTTP request. Implemented through Go's standard library, the multi-part package simplifies the creation of these requests by managing boundaries that separate data segments and facilitating the inclusion of file and form field data. By understanding and utilizing these components, developers can seamlessly send files via HTTP, making the process both efficient and accessible.
No links available for this lesson.
One common operation when it comes to working with HTTP is the ability to send up files. This is often done within the web browser, such as uploading a profile picture, or storing a file on a remote server, such as a video file. However, oftentimes when it comes to CLI applications, the ability to upload files is needed as well.
Currently, we know how to send data up to the HTTP bin service, through the use of a POST request. We've managed to achieve this by encoding our data in various different ways, such as as an HTTP form, or by encoding it through the use of, say, a JSON encoder. So let's go ahead and actually try and send up a file through this approach.
The file we're going to send up is the words.txt
file, back from when we were counting words at the beginning of this course, which feels like a long time ago. For this example, let's go ahead and send up the words.txt
file through the HTTP body field. To do so, let's go ahead and load it in first of all. So file error
. And we can actually just go ahead and ignore the error just to keep things a little concise. And we'll do the os.open
function. We don't need open file for this one. And we'll do dash . / words . txt
. Then let's go ahead and add a deferred statement to close the file, just so that we're tidying up after ourselves. And we can replace the call to HTTP.nobody
with a call to the file instead.
Now, if I go ahead and run this code as follows, you can see after a short while, this is because I'm on a VPN, which is hiding my IP. You can see that we actually don't have any files being sent up, but the data of the file is. Unfortunately, this isn't what we want. In some cases, sending up the files contents as data is okay, but we really want this to come through as an actual file, which is often handled differently when it comes to HTTP servers. For example, files may allow for a much greater amount of data to come through, whereas the data field may be more restrictive.
So how can we get this to actually come up as a file? Well, in order to do so, when it comes to HTTP, you need to send up files through what's known as a multi-part request. This multi-part request, or multi-part / form data, is slightly different to any other data that we've actually sent up to the HTTP service. It allows you to mix different data types, such as text, images, JSON, inside of a single request, and is essential when you need to upload files, as it effectively handles binary data. By the way, I will link to this article in the description down below, just so that you can read it in your own time if you want to.
The way that multi-part form data works is rather technical under the hood. However, it defines something called a boundary, which is a unique string that separates each part of the payload. As you can see here, the boundary is defined as follows, and then you can send up different forms of form data. Whether it's a standard HTTP form field, such as name, username, whether it's a file, such as form data, which is a profile picture, and has the binary data of the actual JPEG image, or whether it's some JSON as well. In our case, we just want to use the multi-part form data in order to send up some binary data, which is going to be the contents of our words.txt
.
Whilst the words.txt
is a simple approach to do this, other things may be sending up images or even video files, which you can also do using this transport as well. Therefore, in order to create a multi-part form, we can actually go ahead and use the multi-part package of the Go standard library, which provides us a multi-part writer, which is used to wrap an IO.writer
and will perform all of the boundary writing for us. Therefore, we need to go ahead and actually define an IO.writer
, which in this case can just be a simple bytes.buffer
, which we can do as follows. And we'll make sure to take the address of this as well. Then we can replace the call to the file with a call to the buffer instead, and let's go ahead and pass this into our writer.
Okay, with that, we can go ahead and capture the return value of this function, which will be our actual multi-part writer, and we can begin writing data to it. However, we can't actually just use the write method of the IO.writer
. Instead, we need to use the either create form field or create form file. We could also use the create path method as well, which will allow us to pass in anything that isn't a file or a form field, such as some JSON data, YAML data, anything really that we may want to pass up. In our case, we want to use the create form file method, which accepts two parameters, a field name and a file name, and will return an IO.writer
that we can write to, as well as an error in case something goes wrong.
So let's go ahead and set this to be words
, and we'll set the file name to be words.txt
, which is the file that we're opening up, and we'll go ahead and actually capture the, I guess, file writer. We'll just call this W
for the moment. And in this case, we're going to go ahead and ignore the error. However, normally you would actually want to handle this, obviously.
Okay, now we can actually go about writing our file to this IO.writer
. To do so simply, let's go ahead and just use the IO.copy
command, copying in the, copying to our writer from our file. Then all that remains is to go ahead and actually close the multi-part writer, which we can do as follows, using the close
function.
Now, if we go ahead and run this code, let's take a look at what happens. Unfortunately, our files is not populated. However, if we take a look at the data field, you can see this time we have the random boundary string, as you can see, as well as actually the form field data, which looks very similar to the example given on the following website. If we take a look at this, you can see we have form data, which is the name of words
, and the actual file name is being passed as well. Then we've got the content type, which is an application / octet-stream
, and the actual content of the file, which is, it says none, but it's one, two, three, four, five. Then we have some new line boundaries.
So as we can see, our data is coming through, but it's not being interpreted by the server as an actual file. In order to do that, we need to go ahead and set a content type header, which fortunately, the multi-part writer makes it pretty easy to do. In order to do so, let's go ahead and call the header.add
method, and we'll go ahead and set the key to be content-type
. Then for the actual header value, we can access this from the writer of the form data content type
method that contains the content type for an HTTP multi-part / form data with this writer's boundary.
Now, if we go ahead and run this code again, this time we should see our file field be populated, which it is. We can see we have the words
file, which contains the contents of our words.txt
file. As you can see, Go makes it incredibly easy to be able to send up files through HTTP, which if you are writing by hand can sometimes be quite difficult to do. As you can see, the content type header has the multi-part / form data, as well as the actual boundary for all of the different parts that we may be sending up.
In addition to sending up files, we could also send up other data using this multi-part writer. So let's say we want to set up a form field of username, which returns us another writer, which we can capture as follows, and we can go ahead and write the username of say Thanos or Sephiroth, or let's go ahead and actually choose a hero. Let's choose Cloud. Let's make sure we actually do this after we close.
Now, if we go ahead and run this code, we should see our username appear in the actual form values as well, which we can see here, username: Cloud
. We could also get rid of the new line by using just fprintf
. And if we go ahead and run this, we should now just see the actual username come through without a new line character.
With that, we've taken a look at how we can actually send multi-part form data to an HTTP service through the use of the multi-part package found in the Go standard library. As I mentioned before, Go makes it incredibly easy to be able to perform HTTP requests and the various different types of requests that you need to use, especially when it comes to sending up things such as form data, JSON encoding, or even files themselves.
In the next lesson, we're going to go back to taking a look at how we can actually test some of our code. Now that we have all of this ability to send up HTTP requests, how can we actually test them in an automated way? So in the next lesson, we're going to take a look at how we can do that, making use of the HTTP test package in order to test HTTP requests.