Please purchase the course to watch this video.
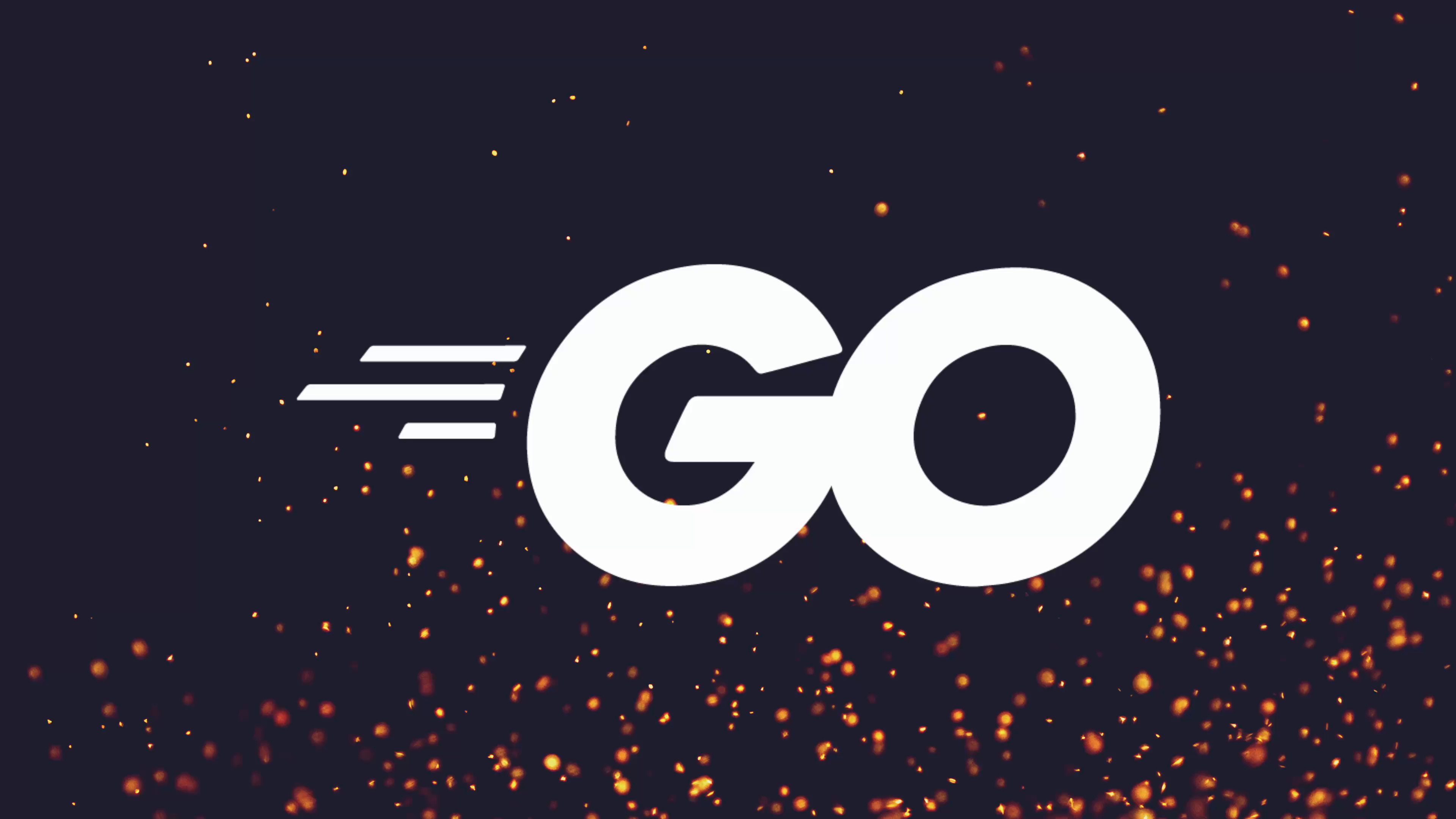
Full Course
File locking is a crucial technique for ensuring data integrity in multi-process applications, particularly when multiple instances of the same program may attempt to read from or write to the same file concurrently. By implementing a file lock using the syscall
package, applications can prevent race conditions and duplicate entries when accessing shared resources. This approach functions similarly to mutexes in concurrent programming, blocking subsequent processes until the lock is released. Effective use of file locks not only enhances reliability but also requires careful handling to avoid deadlocks. Overall, mastering file locking techniques in Go is essential for developing robust applications that maintain data consistency.
No links available for this lesson.
In addition to using both lock files and PID files in order to prevent concurrent applications from running at the same time, by exiting early if the file exists, and if it's not owned by the current process, or if the process owning it doesn't actually exist, in some cases you may instead want to lock an actual file, similar to how we would actually use a mutex when it came to concurrent code.
To short I mean, here I have a really simple Go application. This application accepts a single argument which is passed into a number. Then the application creates a file called numbers.txt
if it doesn't exist already, or opens it up to append to if it already does. Then this code gets the last number in this numbers.txt
file, converts it into an integer, and then iterates over the number of times that we pass in, writing an incrementing value to it.
To show this in action, if I go ahead and remove the numbers.txt
and go ahead and actually just create it so we can tail it out. As you can see numbers.txt
is now empty, and if I open up a new terminal window and use the tail -f
command, which will allow us to actually follow along with the numbers.txt
as it's written to, then if we go ahead and run the code, passing in the number 10, you can see it's writing these files to the numbers one by one.
Then once it reaches the ninth number or the tenth number, it should exit. If I go ahead and run this code again, passing in the number 5, you should see it continues from the last number and adds 5 more numbers to it, up to number 14. You can find a link to this code in the description down below in its current state if you want to develop along at the same time.
Whilst this code works, if we go ahead and actually run multiple instances of it at the same time, you'll see it runs into a bug. As you can see, the numbers are starting to be written out in a duplicate way. Since I'm running two applications, it's writing the same number twice. Before one application quits, it then starts to count up correctly.
Therefore, let's go ahead and actually solve this bug by making use of a file lock, in order to prevent two applications from reading and writing to this file at the same time. In order to do so, we need to make use of the syscall
package, which we actually looked at in the last lesson when it came to sending a signal to our process ID.
This package contains an interface to low-level operating system primitives, and allows you to make system calls when it comes to Unix-like operating systems, which can be very useful for certain situations. Just to be advised, this is a very low-level package and so not all of the features are available for every operating system. For example, the function we're about to look at isn't available on Windows systems, so the function we're about to look at is only available on Linux and macOS and may be available on some other Unix-like systems as well, but it won't be available on Windows. So if you are using Windows, I recommend using Windows subsystem for Linux for this lesson.
In any case, if we look at the documentation for the file lock function, or flock
function, you can see there's not very much. This is one of the issues when it comes to using the syscall package, so instead we actually need to go ahead and look at the Linux system documentation, or the Linux man pages.
Here you can see we have the flock
function, which has the number 2 after it. This means it accepts two arguments. If we scroll down, we can take a look at what those two arguments are. The first is an integer with the name fd
, which stands for file descriptor when it comes to Linux systems. The second value is an operation, which is the argument to specify the locking operation we want on the file. In this case, we have three options we can choose.
LOCK_SH
, which places a shared lock, meaning more than one process may hold a shared lock for a given file at a given time.LOCK_EX
, which places an exclusive lock - this means only one process may hold an exclusive lock for a file at a given time, and is the function we want.LOCK_UN
, which removes an existing lock held by the process.
Let's go ahead and make use of it. As a note, this function will block until the lock becomes available, which is what we want in our current setup. In some cases you may want this to not block and then exit early, which is what we saw when it came to file locking earlier.
In any case, to use this function, we first need to go ahead and import the syscall
package, which we can do as follows:
import "syscall"
And this needs to go here. Then in order to use it, we're going to want to obtain the lock just after we open our file, before we obtain the last line. Therefore, to do so, let's go ahead and call the syscall
package, passing in the flock
function, which as you can see, takes the file descriptor as the first argument and the how as the second, which is the operation. It also returns an error.
Therefore, in order to obtain the file descriptor of our file, we can go ahead and call the fd
method of it, which returns an uint pointer. In our case, we need to cast it to an integer in order for it to work with the flock
function. Next, with our file descriptor passed in, we then need to pass in an integer representing our how. However, as you can see, there is no integer value given here.
Fortunately, there is a constant provided by the syscall
package, which will have the same name as the actual lock constant defined here. In this case, LOCK_EX
or LOCK_EX
, which stands for lock exclusive. As an aside, this function can fail, so let's go ahead and capture the error and handle it. We can do:
error := syscall.Flock(fd, syscall.LOCK_EX)
if error != nil {
log.Fatal("Could not lock file:", error)
}
And we'll just do a log.Fatal
. Fatal line could not lock file. And we'll just go ahead and error this out, print out the error. We'll give a colon just so we give some separation between the error and our message. With that, we should now be locking the file exclusively before we then start processing it.
The last thing we need to do is to make sure that we unlock the file once we're done. To do so, let's go ahead and add it in after we've finished writing to our file. Again, we can do an error capturing the syscall.Flock
function, passing in our file descriptor cast to an integer. And this time using the syscall.LOCK_UN
constant, which is used for unlocking the file.
Then let's go ahead and do the if error check as we always need to do:
error := syscall.Flock(fd, syscall.LOCK_UN)
if error != nil {
log.Fatal("Could not unlock file:", error)
}
Yes, yes, yes. And with that, our file lock should now be in place. Let's go ahead and reset our state just so that we can see this is the case. And we'll do:
rm numbers.txt
We'll go ahead and remove the numbers
as follows. Now, if I go ahead and tail these, let's do a split screen this way. I'll make my face small as well. Let's go ahead and actually touch the numbers.txt
so they exist and they should do.
Okay, let's go ahead and add a tail to them. I'm also going to just go ahead and make this smaller so we can have all of it on screen at the same time. Okay, perfect. So now we're tailing the numbers. If we go ahead and run them and we'll pass in, say, 20 this time, as you can see, they're being written out.
But if I go ahead and run this code again and pass in another 20, these numbers won't be written out until the first process has unlocked the file, which we should see as it comes to number 20. Maybe 20 was too many. In any case, once it hits number 19, we should then see the other file pick up, take on and continue writing the rest whilst this one exits, which we can see is the case.
As you can see, adding in a file lock in order to prevent multiple applications from being able to access the file at the same time is very similar to the concept of using a lock file or a PID file. However, the difference here is that it works more like a mutex, in that it will block the file from being able to proceed until the file is unlocked.
This, in some ways, can be much more beneficial depending on your use case, as you can have a mutex, as you can have a file acts as a sort of mutex, which means the other process will eventually run once that file is unlocked. However, just like with mutexes, you need to be careful to make sure you don't end up in a deadlock state, so I would recommend only ever using one at a single time, and don't use too many in your code.
In any case, that covers how we can use files when it comes to locking our application, be it an exclusive lock through a lock file or a PID file, or locking a file through the use of a system call. This comes in handy when it comes to performing more data integrity related tasks, ensuring that your applications aren't competing for a file resource.
In the next lesson, we're going to start taking a look at networking when it comes to Go, making use of some of the net primitives, then moving on to HTTP, and we'll combine the two files and networking in order to then send files through HTTP later on. As I mentioned before, this code should be available in the lesson description down below, and if you want to, I recommend trying to abstract this actual file locking into its own lock type that we saw at the end of the last lesson. Once you've given that a go and feel confident that you can abstract these concepts, you're well on your way to being a systems programmer when it comes to writing Go code.