Create an account to watch this video
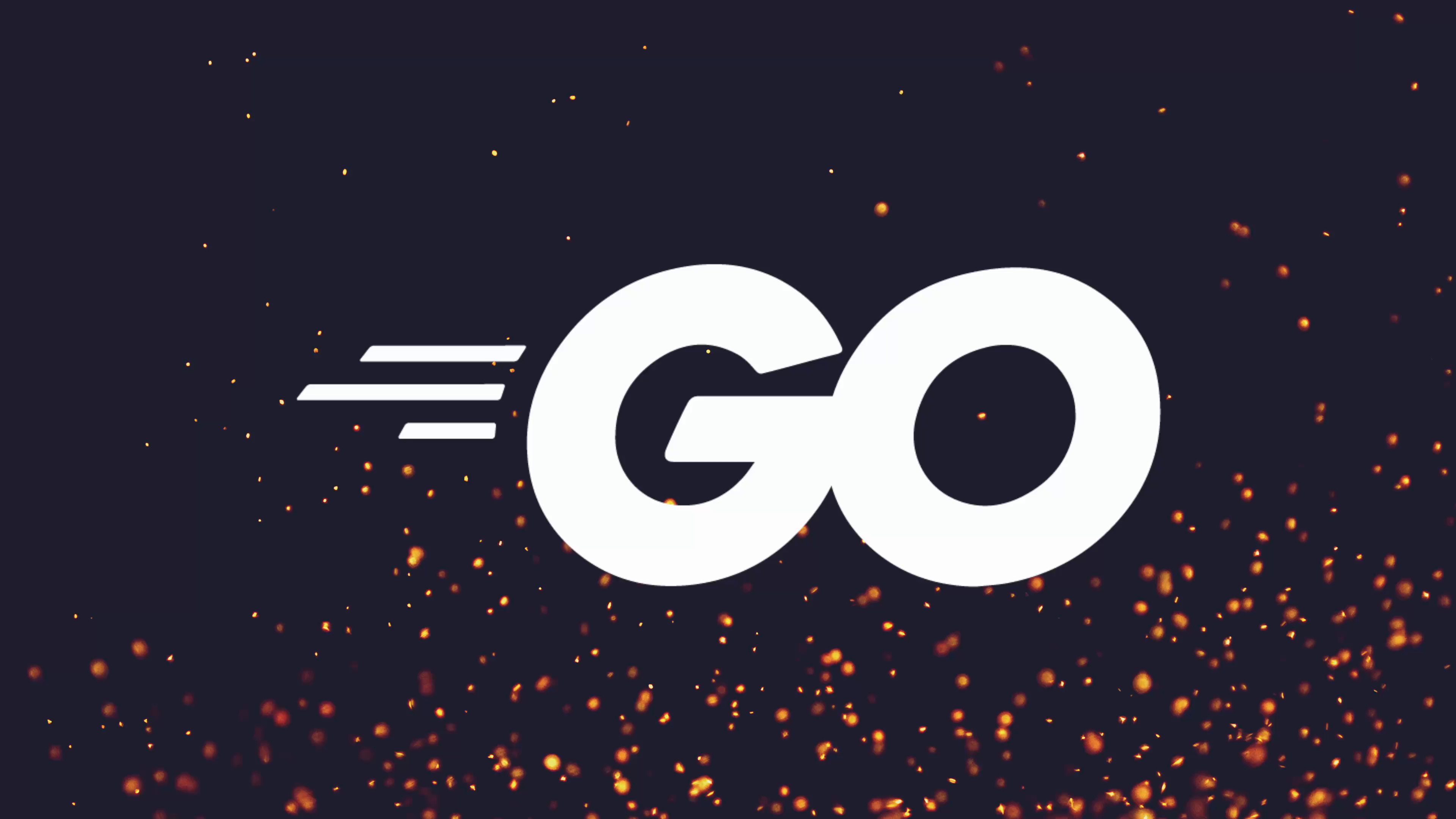
Full Course
Error handling in command line applications is crucial for providing users with clear feedback when something goes wrong. Utilizing standard error (stderr
) instead of standard output (stdout
) for logging error messages is essential to maintain data integrity and assist in automation. While applications may print out results and errors to the console, mixing these outputs can lead to confusion, particularly when piping results to other processes or files. The use of the log
package in Go simplifies error logging, as it automatically handles writing to stderr
and includes useful features like exit status and timestamps. By distinguishing between normal output and error messages, developers can create more robust applications that inform users effectively without obscuring important diagnostic information.
In this lesson, we're going to look at the second approach when it comes to handling errors, which is to inform our users that something went wrong. This is a pretty obvious approach when it comes to error handling, but it actually comes with a couple of caveats when building command line applications that we need to be aware of.
In order to explain what these caveats are, let's begin by adding in a log output in our error handling blog. Here we'll use the Println
function of the fmt
package to let the user know what exactly went wrong. First passing in the string of failed to read file
, followed by printing out the actual error. Now if I go ahead and change this file to be one that doesn't exist, and go ahead and run the go run
command as follows, you can see we're now letting the user know that an error occurred.
Whilst this is useful to let the user know something went wrong, we're actually doing this incorrectly. This is because when it comes to CLI applications, we shouldn't actually print errors out the same way we would when it comes to writing our application output. This is because the Println
function of the fmt
package writes its output to a file stream known as "Standard out, or stdout
.
Standard out, or standard output, is actually one of three different file streams that every process has access to by default. With the other two being "standard input", which is used by the application to read input data, and "Standard Error", which is used by the process to write error messages or diagnostics. We'll take a look at what standard input is later on in this course, however for this lesson let's instead focus on both standard output and standard error, specifically the differences between the two and why you would want to use one instead of the other. The general rule of thumb is that you should use the standard error stream for writing any error messages or diagnostics, and only use the standard output stream for any of the main output of your application code. By doing so, it helps to prevent both your diagnostics and error messages from mixing in with the results of your application, allowing you to not only easily differentiate between the two, but it will also prevent any potential data integrity when piping to other processes, files, or even other systems.
To show why mixing the two can be an issue, here I'm loading in a large file with a lot of words, which if I run the counter
command, will print the number 3000
. If I go ahead and pipe the result of this command into the following awk
command, which will divide the number coming from the counter
command by 80
in order to estimate the number of minutes it took to write this file, if I go ahead and run this command, you can see it returns the value of 37.5
. However, if I go ahead and change this file to one that doesn't exist, whilst we're still logging out errors to stdout
, then if I go ahead and build this code, followed by running it again, piping it into the awk
command, you can see this time it produces the number 0
.
This is not only a problem because the result is incorrect, but we also no longer have the error message telling us what went wrong. This can be especially bad if you happen to be piping the output into a results file, such as follows. If I go ahead and print the results of this file, you can see we now have a line telling us that the number of minutes was 0
. This is an issue as we have no idea that the command actually failed.
For example, if I go ahead and change this again to be lotsofwords.txt
and run the following go run
command again, piping the results to a file named results.txt
, if we go ahead and check the file, we can see again we've got the correct number of minutes. However, if I accidentally make a typo of lotsofwords.txt
and run the same code again, this time whilst we do get an exit status of 1
, if we go ahead and print the results of the file, you can see we get the number of minutes still 0
.
Whilst we could estimate that this value was caused by an error, we don't actually know. Especially if we happen to run this code against an empty file, like I have here, which again produces the same value. This is a bit of a problem, especially when it comes to things such as automation.
Therefore, to resolve this, let's instead go ahead and write our error message out to standard error. In order to do so in Go is actually rather simple. Rather than using the Println
function of the fmt
package, we can instead use the Fprintln
function, which takes as its first parameter an io.Writer
. We'll take a look at interfaces in more detail later on throughout this course. However, for the moment, you can understand that the io.Writer
interface is any type that has the following Write
method, which means we're able to write data to it.
One such type that conforms to this interface is the os.File
type, of which both os.Stdout
and os.Stderr
conform to. Therefore, we can pass the os.Stderr
, which represents standard error in our process, to this fmt.Fprintln
function, followed by then printing out our log line, letting the user know what went wrong.
Now if I go ahead and change this file to again point to a file that doesn't exist, such as follows, then go ahead and run this code using the go run
command, you can see we get the exact same output as we did before. However, it may not seem like it, but it is actually slightly different. This is because, by default, both standard out and standard error are both printed to the console in the terminal. To show what I mean, if I go ahead and make the following changes: change setting the file name to be a variable called noexist.txt
, then making use of this file name and also printing it out to standard out using the Println
command, such as follows, reading from filename
. Now if I go ahead and run this code again, you can see both of these are being printed to the console.
However, if I go ahead and run the following command, piping the output of standard out to a file named output.txt
, this time you'll see that only the standard error stream is being printed out. And if we take a look at the contents of the output.txt
, you can see that it contains the contents of our fmt.Println
statement. This is because, by using this syntax, we've managed to forward the output of std out
into a file called output.txt
. In fact, we can actually do the same thing when it comes to standard error. If I go ahead and load the same command as before, however, I make the following changes of using to greater than
and piping it to a file named errors.txt
. This time you'll see that we get the standard output stream printed to the console. But if I take a look at the errors.txt
file, you can see it contains our error messages.
You may have noticed in this command that it's very similar to the way that we forwarded std out
. However, instead of just using the greater than symbol, we're using it prefixed with the number 2
. This is because a process by default will have three file descriptors, and the file descriptor with the id number of 2
is by default standard error. These file ids are 0
for standard input, 1
for standard output, and 2
for standard error. You can actually change these file descriptors and their ids. However, I wouldn't ever recommend doing so as it can easily cause some problems. By being able to forward both standard out and standard error, we can actually do some interesting things. For starters, we can forward both of these out into their own separate files as follows. Let's go ahead and set these to be number 2
so that we're not overwriting the existing ones. This prevents any output from entering the console, but at the same time we get an output to logs.txt
and an errors.txt
. This is incredibly useful when it comes to forwarding different logs to different positions, so that you're able to easily see the errors that have occurred with your process without having to sift through the actual output. Not only this, but it also means you could pipe the results of your errors to another process, or pipe the results of your actual application to another process as well.
With these changes made, let's go ahead and delete the reading from file that we put before, and take a look at how it now works when it came to our calculation function in order to work out the number of minutes that it would take to write a file. Again, we're going to go ahead and run this against the no existing file, which this time, rather than producing an actual calculation of 0 minutes
, just produces the error that we saw before, letting the user know that something went wrong and a calculation shouldn't be generated from it. By writing errors to the standard error file stream, it's now safe to use this code in things such as automation, whilst also keeping the ability to inform the user what went wrong.
However, this can be a little tedious to do over and over again. Fortunately, as we've seen before, the Go standard library provides functionality to make this pretty easy to do without needing to remember to both os.Exit
and to pipe into the os.Stderr
. This is provided by the log
package in the standard library, which provides the following three methods we can use in order to both exit and to print to standard out. These include the Fatal
function, which is very similar to the Println
function, the Fatalf
function, which is similar to Printf
, and the Fatalln
, which is equivalent to Println
. In addition to being equivalent to their following print statements, they'll also call the os.Exit(1)
, which makes it much more concise for our own use case.
To show how this works, let's go ahead and import the log
package and call the Fatalln
function, passing in the same string we were using before in order to inform our user. If I then go ahead and run this code, you can see this time we get our Println
statement, as we had before, of failed two read file:
with the actual error that we encountered, as well as the exit status of 1
, showing us that the Fatalln
function is also calling the Exit
function of the os
package. One thing you may notice is that we also get a timestamp added to the prefix of our log line. This may or may not be desirable depending on what your application is, but there is a way to disable it. Before we look at how to do that however, let's first make sure that this is actually being printed to Stderr
, by first removing the two errors.txt
files that I have, perfect, before now running the following go run
command, piping the output of Stderr
into errors.txt
. Now if I print out the contents of errors.txt
you can see it has our actual log line, as well as no longer printing this to the actual console. This makes the log
package much more preferable when it comes to actually logging out to Stderr
, as it's much more concise than remembering to use the os.Exit
line.
As I mentioned, if you want to remove the actual timestamp itself, you can use the setFlags
method of the actual log
package. This allows you to set the output flags for the standard logger, which includes a number of flags such as ldate
, ltime
and so on. Personally, I like to get rid of all of these when it comes to my CLI applications, so I just set the bit flag for this value to be 0
. Now if we go ahead and run this code again, you can see we no longer have the date and time being prefixed in our log message. With that we've taken a look at how we can actually communicate errors to our user when it comes to writing CLI applications, by doing so safely by writing to os.Stderr
.
That wraps up the end of this lesson. In the next one we're going to improve our algorithm a little further, by ensuring that we can read from files of any type of size. I look forward to seeing you there!