Please purchase the course to watch this video.
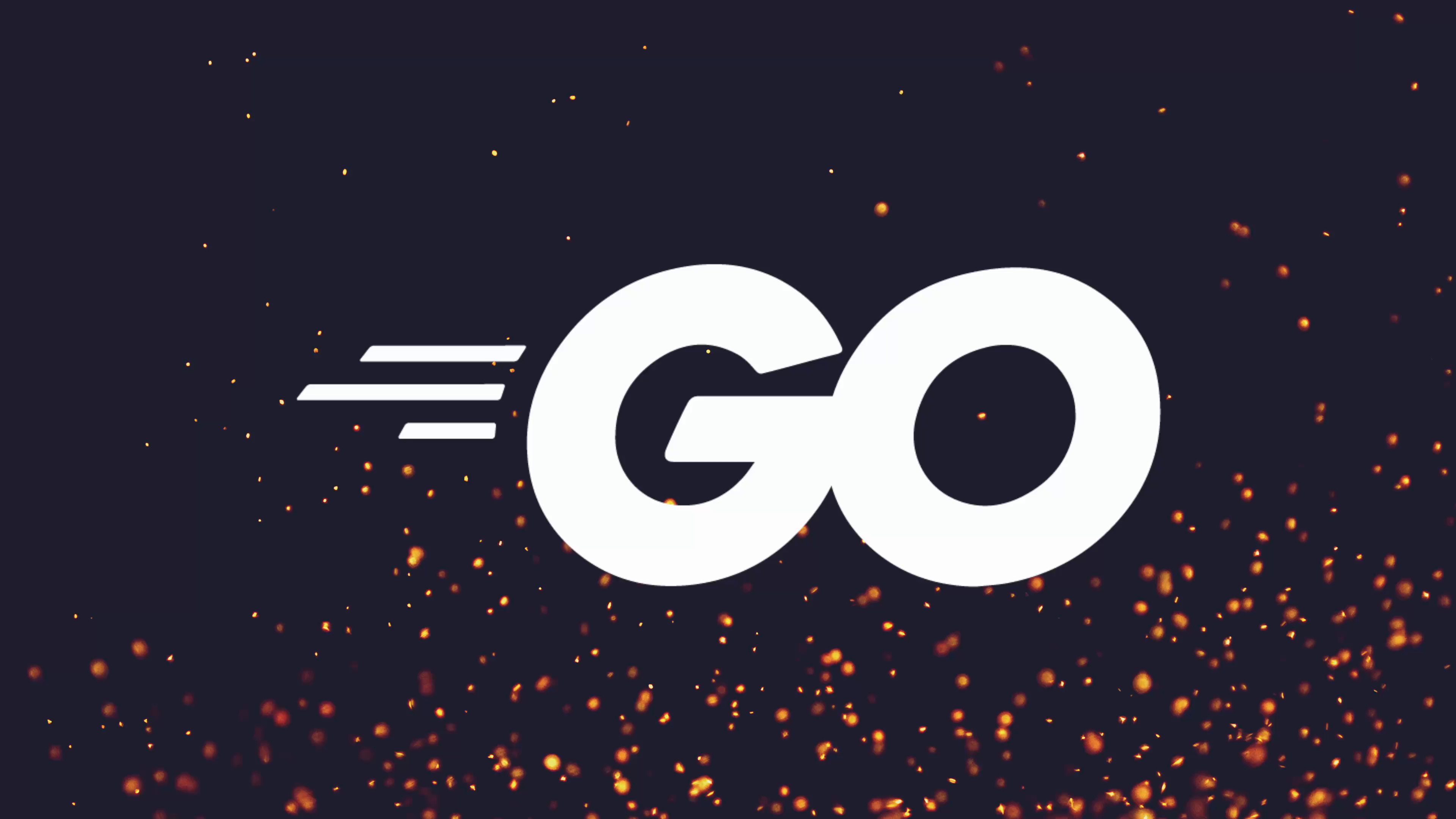
Full Course
Incorporating methods into data types enhances code reusability, but challenges arise when unnecessary parameters, such as a filename, complicate the interface. A common issue occurs when the function requires a filename, even when it may not be needed, leading to awkward conditional checks for empty inputs. A more elegant solution involves using variadic parameters, allowing the function to accept a flexible number of input strings, including none at all. This simplifies the code by transforming the filename parameter into a slice of strings, enabling seamless handling of zero or more filenames without compromising the function's usability. This approach not only streamlines the code but also maintains clean, user-friendly interfaces, paving the way for further enhancements, such as combining counts in future lessons.
No links available for this lesson.
In the last lesson, we took a look at adding a method into our Counts
type, in order to be able to print the various properties to the console.
This gave the benefit that we were able to reuse this function across multiple different places, on line 31, line 35, and line 39.
However, one of the issues that I currently have with this function is the fact that we have to pass in a file name, even when we don't want one. And in fact, it actually caused an issue with our individual tests, and we ended up needing to use a conditional check to see if the file name was empty in order to ignore it from being printed out.
This to me feels like a bit of an exception when it comes to our code, as there could potentially be the situation where an empty file name may exist, although it's hard to imagine one actually happening. In any case, there's now an exception to the logic of our code, in that we're accepting a file name, but if it's empty, we won't actually print it out, which to me feels a little strange.
For example, if we happen to pass in an empty space, or even a new line, this file name will still be printed out, even though it's kind of the same as printing out an empty file name. However, we're making a distinction for this situation, but we're not making an exception for the others.
Of course, we could add an exception by first using the strings.TrimSpace
function we've seen before. However, in that case, it starts to feel like we're adding in specific exceptions for this function, when there may instead be a better way to handle the fact we don't always want to pass in a file name.
Therefore, let's take a look at another approach, which is to make use of something called a variadic parameter.
We've actually seen variadic parameters already. In fact, the fmt.Println
function makes use of one already. As you'll notice, when we call this function, we're able to pass in multiple parameters to it. However, the actual parameter type that it accepts is an any
, with a ...
prefix. This:
func Println(a ...any)
This ...
prefix specifies that you can pass any amount of parameters in, such as:
fmt.Println("foo", "bar", "wibble", "wobble")
As you can tell, we're passing in four parameters to this function, despite it only suggesting one parameter as an input. This is because a variadic parameter allows you to pass different numbers of inputs to it—such as four, three, one, or even zero. The fact that it allows you to pass zero in is what makes it appealing to our use case.
Therefore, let's go ahead and change our file name parameter and turn it into a variadic parameter, such as follows:
func (c Counts) Print(filenames ...string)
By doing so, it means we can now go ahead and change the code on line 35 from passing in an empty string as the filename to now passing in no filename, which to me feels like a much better interface.
Now that we've done this, we need to update our implementation.
Previously we had:
if filename != "" {
fmt.Fprintf(w, " %s", filename)
}
But now, the parameter filenames
is a slice of string ([]string
). So we interface with it as a slice.
To print all the file names (if present):
for _, filename := range filenames {
fmt.Fprintf(w, " %s", filename)
}
With that change made, if we go ahead and run our code now using:
go run main.go words.txt
You’ll see everything works the same. And if we use stdin:
cat words.txt | go run main.go
Again, it works as expected.
However, if I go ahead and run the tests, they now fail. The failure is due to a space being printed before the newline character, which was not present in our test expectations.
If we take a look at the test code, you'll see our test cases are still passing in a filename as a string. We're performing the old behavior, even when we don't want to pass a filename.
To solve this:
- We want the ability to pass zero or more file names
- Variadic parameters cannot be used inside structs
- Instead, we use a slice of strings
Refactor the test case struct:
type Inputs struct {
Counts Counts
FileNames []string
}
Then update test cases:
Inputs{
Counts: Counts{Lines: 1, Words: 5, Bytes: 24},
FileNames: []string{"words.txt"},
}
Or, for no filename:
Inputs{
Counts: Counts{Lines: 20, Words: 4, Bytes: 1},
// FileNames omitted or set to nil
}
Now we need to pass the slice to our method.
The issue is:
c.Print(tc.Input.FileNames) // invalid
Go requires us to unwrap the slice into a variadic parameter using ...
:
c.Print(tc.Input.FileNames...)
This allows a []string
to be passed into a ...string
parameter.
Now, if we run the tests:
go test
They pass 🎉
✅ Summary:
- Replaced
Print(fileName string)
withPrint(filenames ...string)
- Cleaned up the interface—no more needing to pass an empty string
- Refactored tests to pass slices instead of strings
- Used
...
to expand slices into variadic arguments
This is a cleaner, more idiomatic Go interface. And this pattern—variadic arguments—is something we’ll use again.
In the next lesson, we’re going to implement yet another method, this time to add counts together.
See you there.