Please purchase the course to watch this video.
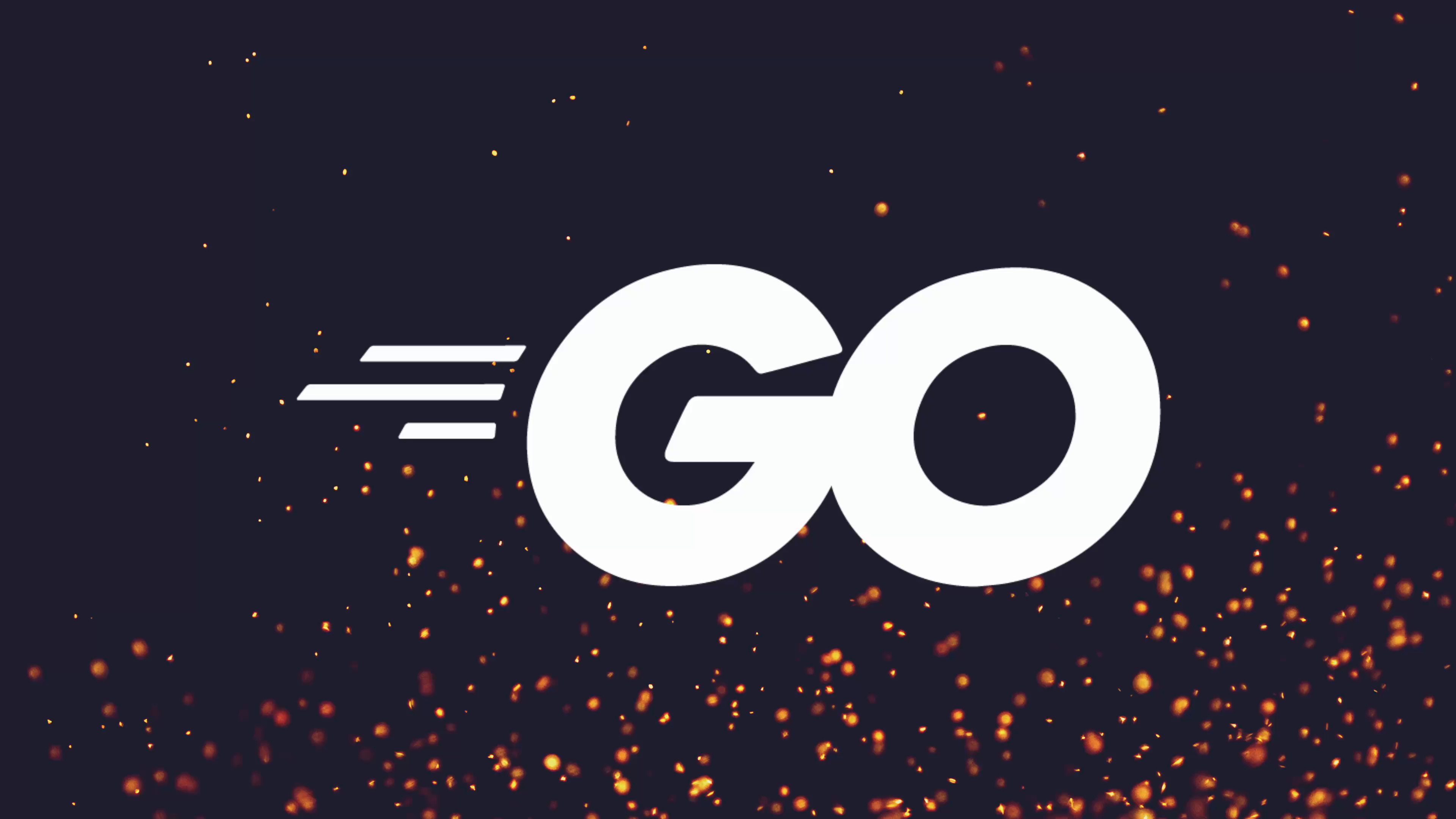
Full Course
Improving code quality through proper package visibility is crucial for enhancing reliability and maintainability in Go programming. By applying visibility constraints, developers can better manage access to struct fields, ensuring that users interact with methods rather than directly modifying properties, thus reducing potential bugs and unexpected behaviors. The lesson highlights the importance of using constructor functions to initialize struct values securely, while also addressing common pitfalls associated with passing parameters incorrectly. By structuring code this way, developers can enforce better practices and create a safer interface for package users, ultimately leading to more stable code during development.
No links available for this lesson.
In the last lesson, we took a quick look at how we can restructure our project code in a way that makes sense — allowing us to export the counting logic or display logic as a reusable package module, while also moving our main.go
into a cmd
directory to maintain our application binary.
Using Package Visibility
In this lesson, we're going to improve our code further by applying package visibility. If you remember back to Go 101, we talked a little bit about package visibility. In that lesson, we mentioned:
All types, functions, and variables defined within a package are package-scoped.
You can make them accessible outside of the package by capitalizing the first letter of their name.
Example: Display Options Package
In our current display
package, everything is publicly accessible:
- This made sense when everything lived inside the
main
package. - Now that we've modularized our code, we can increase reliability by restricting visibility.
Problem with Exported Fields
We previously defined methods like:
func (o Options) shouldShowBytes() bool { ... }
func (o Options) shouldShowWords() bool { ... }
func (o Options) shouldShowLines() bool { ... }
These methods perform validation — if none of the flags are passed, they return true
by default to ensure output is shown.
For example:
# No flags passed
$ go run main.go file.txt
# => prints lines, words, and bytes
# Flag passed
$ go run main.go -words file.txt
# => prints only words
In our print
function, we rely on these methods instead of accessing the struct fields directly.
However, because the fields like ShowBytes
, ShowWords
, and ShowLines
are exported:
- Users might access them directly.
- Users might modify them.
- Go doesn't support constants for struct fields.
This leads to potential bugs and undermines encapsulation.
Fix: Lowercase the Field Names
To solve this, we'll lowercase the struct fields to make them package-private:
type Options struct {
showBytes bool
showWords bool
showLines bool
}
Now, fields like ShowBytes
become showBytes
, and are no longer accessible outside the package.
Benefit of Compilation Errors
After doing this, if any part of our code tries to reference the now-private fields (e.g. ops.ShowLines
), the compiler will throw an error.
This acts as a signal that we should be using the public methods like:
ops.shouldShowLines()
which are still accessible and handle the logic correctly.
The Problem: Setting Fields
Previously, we set flags like this:
showLines := flag.Bool("lines", false, "...")
// ...
ops := display.Options{
ShowLines: *showLines,
// ...
}
But now, since the fields are private, we can’t set them from outside the package.
Solution: Constructor Pattern
Go doesn’t have constructors like C++ or Rust, but we can implement a simple idiomatic constructor:
func NewOptions(bytes, words, lines bool) Options {
return Options{
showBytes: bytes,
showWords: words,
showLines: lines,
}
}
This allows package consumers to initialize the struct safely, without direct field access.
Usage:
ops := display.NewOptions(*showBytes, *showWords, *showLines)
Now:
- Fields are private.
- Struct is initialized correctly.
- Consumers must use the public methods for behavior.
Downside of Constructor with Booleans
There’s one issue:
display.NewOptions(true, false, true)
This is easy to mess up, as it's unclear what each boolean refers to.
Previously, with field assignment, it was clearer:
Options{
ShowWords: true,
ShowLines: false,
ShowBytes: true,
}
What’s Next?
While this new structure improves maintainability and enforces encapsulation, it introduces the potential for argument-order bugs.
We’ll address this next by looking at an alternative approach that improves readability and reduces the likelihood of error.
In the next lesson, we're going to take a look at another approach in order to make our code just a bit more readable.